- What is the Bind Method in JavaScript?
- Why is Bind Important?
- Syntax of Bind
- Practical Use of Bind
- Always remember...
Happy Valentine's day ❤️
Now that we've got that out of the way, let's talk about JavaScript's bind
function.
JavaScript's .bind()
method is a powerful yet sometimes overlooked feature in a developer's toolkit. It plays a critical role in controlling the context (this
) of functions, especially in scenarios where maintaining the context is not straightforward. Let's unravel the mysteries of .bind()
and understand how it can be effectively used in JavaScript programming.
What is the Bind Method in JavaScript?
The .bind()
method creates a new function with the same body and scope as the original function, but where the this
context is bound to a specified object. This means you can set what the this
keyword refers to, regardless of how the function is called.
Why is Bind Important?
In JavaScript, the value of this
within a function depends on how the function is called. It can vary, leading to unexpected behaviors, particularly in event handlers or callbacks. The .bind()
method helps in fixing (or binding) the value of this
, making your functions' behavior more predictable.
Syntax of Bind
The syntax of .bind()
is straightforward:
const boundFunction = originalFunction.bind(thisArg, arg1, arg2, ...);
-
originalFunction
: The function you want to bind. -
thisArg
: The value you wantthis
to refer to in the bound function. -
arg1, arg2, ...
: Arguments to prepend to arguments passed to the bound function.
Practical Use of Bind
- Maintaining Context in Callbacks and Event Handlers:
One of the most common uses of .bind()
is in event handlers and callbacks, where the context can change.
Example:
function User(name) {
this.name = name;
}
User.prototype.sayHello = function() {
console.log('Hello, ' + this.name);
};
const user = new User('Alice');
setTimeout(user.sayHello.bind(user), 1000); // Binds `this` to `user`
Without .bind(user)
, the this
inside sayHello
would not refer to the user
object in the setTimeout
callback.
- Partial Function Application:
.bind()
can also be used for partial application of a function. This means presetting some of the initial arguments of a function.
Example:
function add(a, b) {
return a + b;
}
const addFive = add.bind(null, 5);
console.log(addFive(10)); // Outputs: 15
Here, addFive
is a new function that always adds five to its argument.
Always remember...
Bind creates a new function. Every time .bind()
is used, it creates a new function.
You can't rebind a function. Once a function has been bound, you can’t change its this
context anymore.
Performance considerations shouldn't be forgotten. Avoid unnecessary use of .bind()
inside frequently called functions or loops, as it creates a new function each time.
The .bind()
method in JavaScript is crucial for managing the context within functions, especially in situations where this
does not behave as expected. Mastering .bind()
enhances your ability to write more stable and predictable JavaScript code, essential for event handling and functional programming patterns.
Understanding and utilizing .bind()
effectively is a testament to your depth of knowledge in JavaScript. If you think your JavaScript knowledge is on-point, you should check out our JavaScript Fundamentals certification, which is a perfect way of showcasing your JavaScript knowledge.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
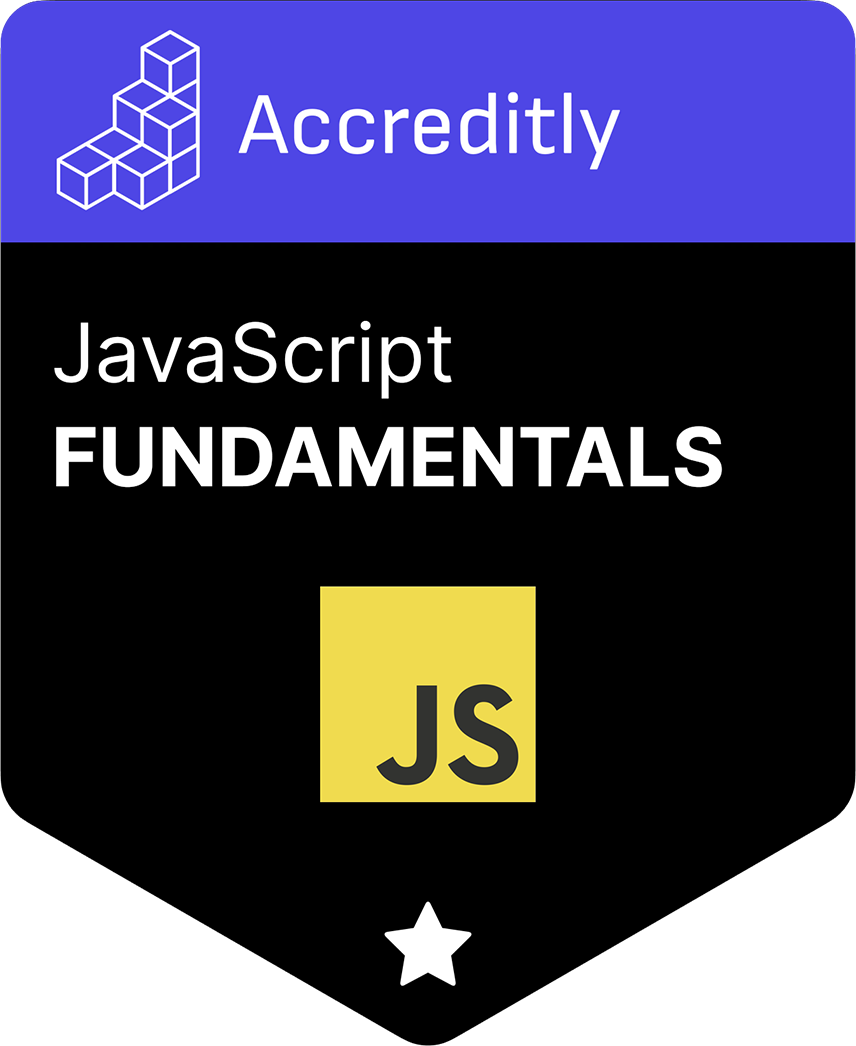