- A New Operator on the Block
- Syntax and Simplicity
- Associativity: Right-to-Left
- Using Exponentiation in Calculations
- Compatibility and Considerations
- A Touch of Caution
- The Deprecated Math.pow()?
- Final Thoughts
In the evolving landscape of JavaScript, every character counts. As we journey through the syntax and semantics of this dynamic language, we occasionally stumble upon small yet significant updates. One such gem is the exponentiation operator (**
), a sleek addition that simplifies raising numbers to a power.
A New Operator on the Block
Before ES2016, if you wanted to calculate exponents in JavaScript, your go-to was the Math.pow()
function:
let square = Math.pow(2, 2); // 4
let cube = Math.pow(2, 3); // 8
Functional? Certainly. But it lacked the directness and elegance that a language like Python offered with its simple **
operator. The JavaScript community took note, and thus the exponentiation operator was introduced.
Syntax and Simplicity
The syntax for the exponentiation operator is straightforward:
let square = 2 ** 2; // 4
let cube = 2 ** 3; // 8
This operator takes the form of two asterisks (**
). It's as intuitive as it gets---no parentheses, no function calls, just pure, succinct arithmetic.
Associativity: Right-to-Left
One important aspect of the exponentiation operator is that it is right-associative. What does this mean for your calculations? The operations are performed from right to left. For instance:
let result = 2 ** 3 ** 2;
// Same as: 2 ** (3 ** 2)
// Result is 2 ** 9, which is 512
Using Exponentiation in Calculations
The true beauty of the exponentiation operator is revealed in its use within more complex expressions. It can make scientific calculations, compound interest formulas, and any other exponential-related computation far more readable.
let earthMass = 5.972 ** 24; // Mass of Earth in kg
let investment = 1000 * (1 + 0.07) ** 10; // Compound interest over 10 years
Compatibility and Considerations
While most modern browsers and environments support the exponentiation operator, it's always wise to check for compatibility if your audience might be using older browsers. Transpilers like Babel can be set up to convert these operators into Math.pow()
calls for wider compatibility.
A Touch of Caution
Just a word to the wise: be cautious with negative bases. The operator can yield different results depending on the parentheses due to the operator's right-associativity.
let positiveBase = (-2) ** 2; // 4
let negativeBase = -2 ** 2; // -4, because it's read as -(2 ** 2)
Math.pow()
?
The Deprecated While Math.pow()
still has its place for certain use cases (especially when dealing with non-integer exponents or when needing to ensure backward compatibility), the exponentiation operator is typically the cleaner and more modern choice for integer powers.
Final Thoughts
With the addition of the exponentiation operator, JavaScript's arithmetic syntax takes a step closer to mathematical notation. It's a small change, but it's one that streamlines our code and aligns JavaScript with other programming languages in terms of arithmetic expression capabilities.
The exponentiation operator (**
) is one of those elegant features in JavaScript that once adopted, becomes indispensable. It's a testament to the language's growth and the community's commitment to improvement. As developers, we revel in these small victories, for they make our code not only more intuitive but a little bit more enjoyable to write.
As always though, remember that readablity is important. When using a language feature that isn't popular or widely known it can often bring about readability issues for other developers, so use with caution, and heavily comment your code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
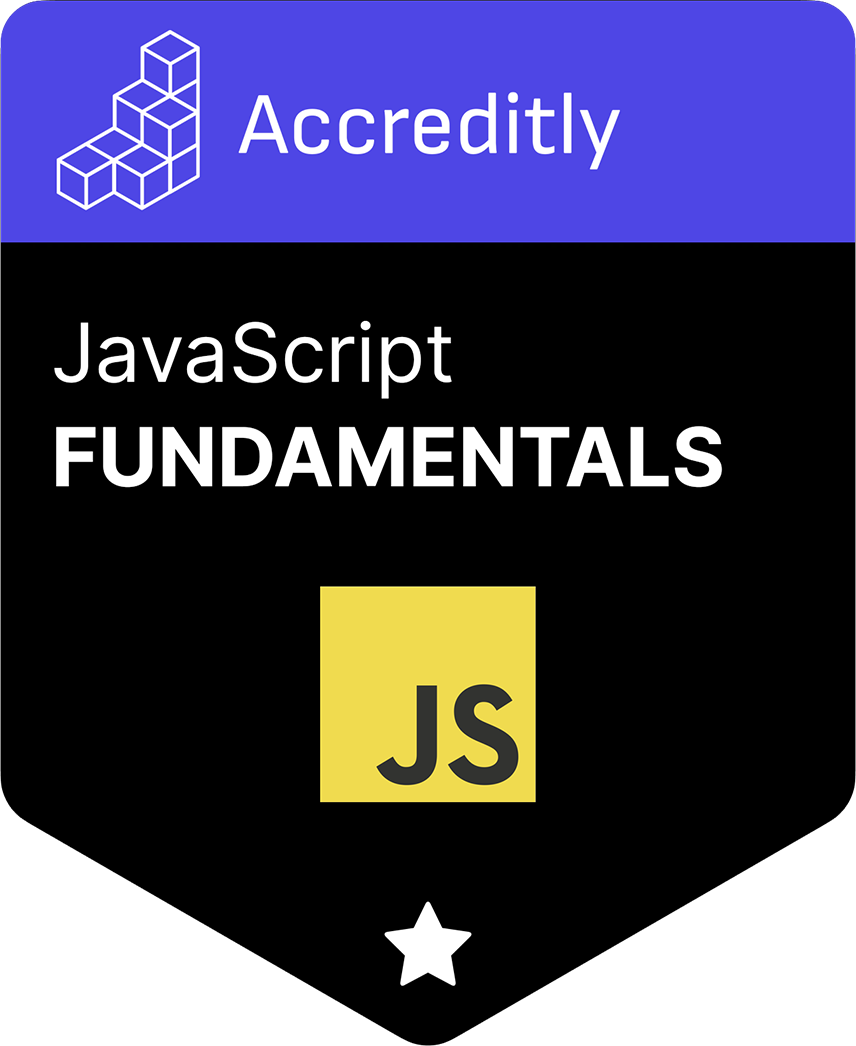