In a world where content is key, protecting your assets is crucial. Watermarking images is one of the most common methods of protecting and identifying digital property. While server-side watermarking is common, there can be benefits to performing this task on the client-side before uploading the image to a server. This article explores how to use JavaScript to apply a watermark to an image on the client-side.
Prerequisites
Before we begin, we need to ensure we have the necessary tools and library. We will be using a library called watermark.js
. This library simplifies the process of applying a watermark on an image on the client-side. You can install it via npm, or include it directly from a CDN.
Include it in your project:
<script src="https://unpkg.com/watermarkjs"></script>
Selecting an Image
Firstly, we need an image to watermark. We will add an input field to our HTML that allows the user to select a file.
<input type="file" id="image-input">
<button id="upload-button">Upload</button>
<img id="preview" src="" alt="Preview">
We also add a button that will trigger the upload process and an img
tag to preview the watermarked image.
Loading the Image and Watermark
Next, we want to use the watermark
object available from watermark.js
to load the image and watermark. To keep things simple, we will use a text watermark.
const imageInput = document.querySelector('#image-input');
const uploadButton = document.querySelector('#upload-button');
const preview = document.querySelector('#preview');
uploadButton.addEventListener('click', () => {
const file = imageInput.files[0];
watermark([file])
.image(watermark.text.lowerRight('Watermark', '48px Arial', '#fff', 0.5))
.then(img => {
preview.src = img.src;
});
});
In this example, we take the selected file and pass it to the watermark
function as an array. We then chain the image
function, where we specify we want a text watermark positioned at the lower right of the image.
The text watermark is added using watermark.text.lowerRight
which accepts four parameters: the text to be displayed, the font, the color of the text, and the transparency level of the text.
Uploading the Image
Once we have the watermarked image, it can then be uploaded to the server. You will need to implement this according to how your server handles file uploads. In the example above, the watermarked image's data URL can be retrieved using img.src
which can be sent to the server in a POST request.
Conclusion
Watermarking images on the client-side is an effective way to secure your images without adding additional load to your server. By using watermark.js
, we can easily add text or image watermarks directly within the browser before sending them to the server.
Always remember to account for client-side manipulations and ensure the integrity of your application by implementing the necessary server-side checks. It would be fairly trivial for a malicious user to skip the watermarking within the browser, so don't rely on it in a production app.
One potential use-case for this is to apply the watermark locally to show the user the image, but the actual 'true' watermarking is done on the server. Doing it this way would save on load if making adjustments (the server would only need to build the final image) and save on round trips, making this faster.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
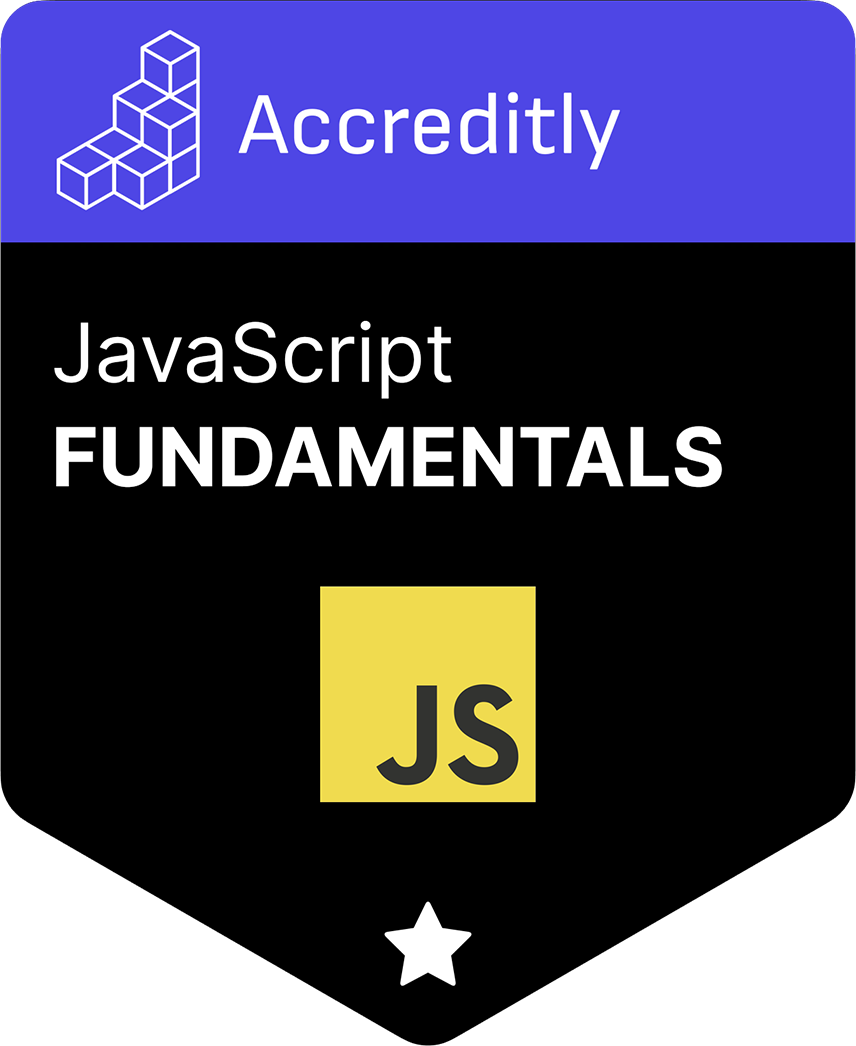