In object-oriented programming in PHP, the final
keyword can be a polarizing topic among developers, especially when it comes to defining classes in reusable packages. While final
can be useful for ensuring a class is not extended in a way that could break encapsulation or violate its intended usage, it can also introduce limitations that affect the flexibility and testability of a codebase. This article examines why final
might be considered problematic for classes in PHP packages, highlighting the challenges and considerations from a software design perspective.
final
Keyword
Understanding the In PHP, the final
keyword can be used in two contexts: to prevent a class from being extended (inherited from), and to prevent a method from being overridden. When a class is defined as final
, it cannot be subclassed:
final class MyFinalClass {
// Class implementation
}
Attempting to extend this class would result in a fatal error.
final
in Packages
Arguments Against Using 1. Inhibits Extensibility
One of the core principles of object-oriented design is the ability to extend existing code without modifying it. This is particularly important in distributed packages where the source code isn't directly controlled by the end user. When a package developer marks a class as final
, they prevent other developers from extending these classes to add or modify functionality, potentially limiting the package's usefulness in scenarios not foreseen by its original authors.
2. Complicates Testing
Testing can become more challenging when a class is marked as final
. For instance, developers often use inheritance to mock or override methods of a class during unit testing. By preventing extension, final
can force developers to use more complex or less intuitive methods for testing the behavior of classes that interact with the final classes.
3. Discourages Contribution
In the open-source community, where contributions and forks are common, final
classes can be seen as a barrier. If developers feel that they cannot easily extend a package to fit their needs, they may choose to fork the repository or look for alternative solutions, potentially fragmenting the community and diluting the pool of contributors.
4. Evolution and Refactoring
Software needs evolve over time, and what might seem like a good use of final
today may not hold in the future. Developers maintaining a package might find themselves needing to refactor or remove the final
keyword to accommodate new use cases, which could break backward compatibility.
final
Arguments for Using Despite these challenges, there are valid reasons why package developers might choose to use final
:
1. Protecting the Integrity of the Code
final
ensures that the core functionality of a class cannot be altered through inheritance, protecting the intended behavior of the class against modifications that could introduce bugs or security vulnerabilities.
2. Encouraging Composition Over Inheritance
Many software design experts advocate for composition over inheritance as a more flexible and less error-prone approach to extending functionality. By marking classes as final
, developers encourage users to use composition instead of inheritance, potentially leading to better software design patterns.
3. Performance Considerations
In some cases, using final
can lead to minor performance optimizations. PHP engines can make certain assumptions about final
classes and methods that allow for more efficient execution.
The use of final
in PHP classes within packages is a nuanced decision that involves weighing the benefits of protecting class integrity against the potential downsides of reduced flexibility and extensibility. Package developers should consider the specific needs and usage scenarios of their libraries, possibly providing extensive justifications in their documentation when they decide to use final
. Meanwhile, consumers of these packages should be aware of these limitations and plan their architecture and testing strategies accordingly. In the end, whether to use final
should be guided by a careful consideration of both design principles and practical implications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
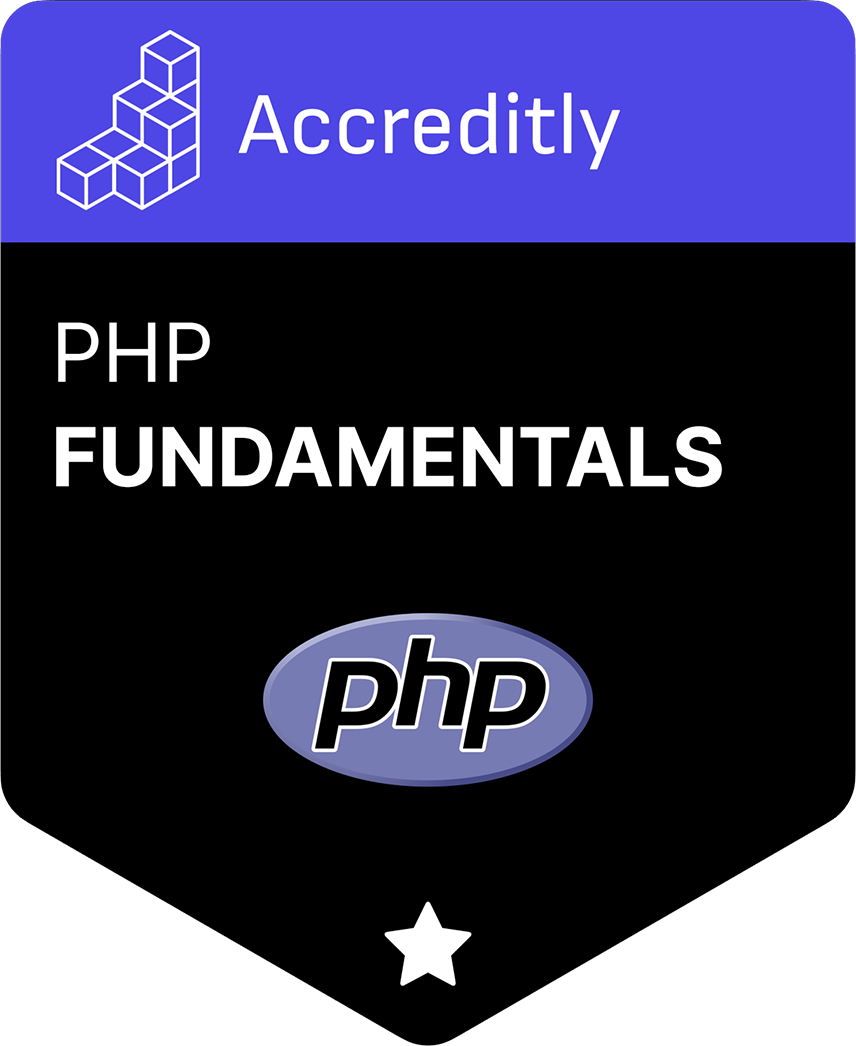