- What is a Blob?
- Creating a Blob
- Reading a Blob
- Creating a Blob URL
- Working with Files
- Converting Between Blobs and Arrays
- Use Cases for Blobs
- Best Practices
- Blobs in a nuteshell
Blobs, or Binary Large Objects, in JavaScript are immutable objects that represent raw data. They can be used for a variety of purposes, such as file operations, image processing, and more. One practical example we've discussed lately is using blobs to chunk uploads in JavaScript.
Let’s dive into the world of Blobs and understand how to work with them effectively in JavaScript.
What is a Blob?
A Blob in JavaScript is an object that represents data that isn't necessarily in a JavaScript-native format. The File interface is based on Blob, inheriting Blob's functionality and expanding it to support user system file operations.
Creating a Blob
You can create a Blob by using the Blob constructor, which takes an array of data and options as parameters. The data can be any JavaScript objects, such as BufferSource, Blob, String, etc.
Example:
const data = new Uint8Array([72, 101, 108, 108, 111]); // Hello in ASCII
const blob = new Blob([data], { type: 'text/plain' });
Reading a Blob
To read data from a Blob, you can use the FileReader
API. This API reads the contents of Blobs and Files and returns data in various formats.
Example: Reading Blob as Text:
const reader = new FileReader();
reader.onload = function(e) {
console.log(e.target.result); // Outputs: Hello
};
reader.readAsText(blob);
Creating a Blob URL
Blob URLs can be created using the URL.createObjectURL() method. This URL can be used as a reference to the Blob object in your application, such as setting it as the source of an <img>
tag.
Example:
const imageUrl = URL.createObjectURL(blob);
document.getElementById('myImage').src = imageUrl;
Working with Files
Since Files are an extension of Blobs, all operations that you can do with Blobs are also applicable to File objects. This is particularly useful for file operations, like reading user-uploaded files.
Example: Handling File Uploads:
const inputElement = document.querySelector('input[type="file"]');
inputElement.addEventListener('change', handleFiles, false);
function handleFiles() {
const fileList = this.files; // fileList is a FileList of File objects
const file = fileList[0];
// Read the file content
const reader = new FileReader();
reader.onload = function(e) {
console.log(e.target.result);
};
reader.readAsText(file);
}
Converting Between Blobs and Arrays
Sometimes, you may need to convert a Blob to an ArrayBuffer for processing binary data or vice versa.
Example: Blob to ArrayBuffer Conversion:
const blobToArrayBuffer = async (blob) => {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = () => resolve(reader.result);
reader.onerror = () => reject(reader.error);
reader.readAsArrayBuffer(blob);
});
};
Use Cases for Blobs
- File Operations: Handling user-uploaded files, such as images, videos, or documents.
- Image Processing: Creating image thumbnails, converting image formats, etc.
- Data Streaming: Streaming large data chunks, like video or audio data.
- Data Storage: Storing binary data locally using IndexedDB or other mechanisms.
Best Practices
-
Memory Management: Revoke Blob URLs when not needed using
URL.revokeObjectURL()
to free up memory. - Error Handling: Always handle errors, especially when reading from or converting Blobs.
- Performance Considerations: Be mindful of performance when dealing with large Blobs, as operations can be memory-intensive.
Blobs in a nuteshell
Blobs are a versatile and powerful feature in JavaScript for handling binary data. Understanding how to create, read, and manipulate Blobs opens up a range of possibilities for web developers in data processing and management.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
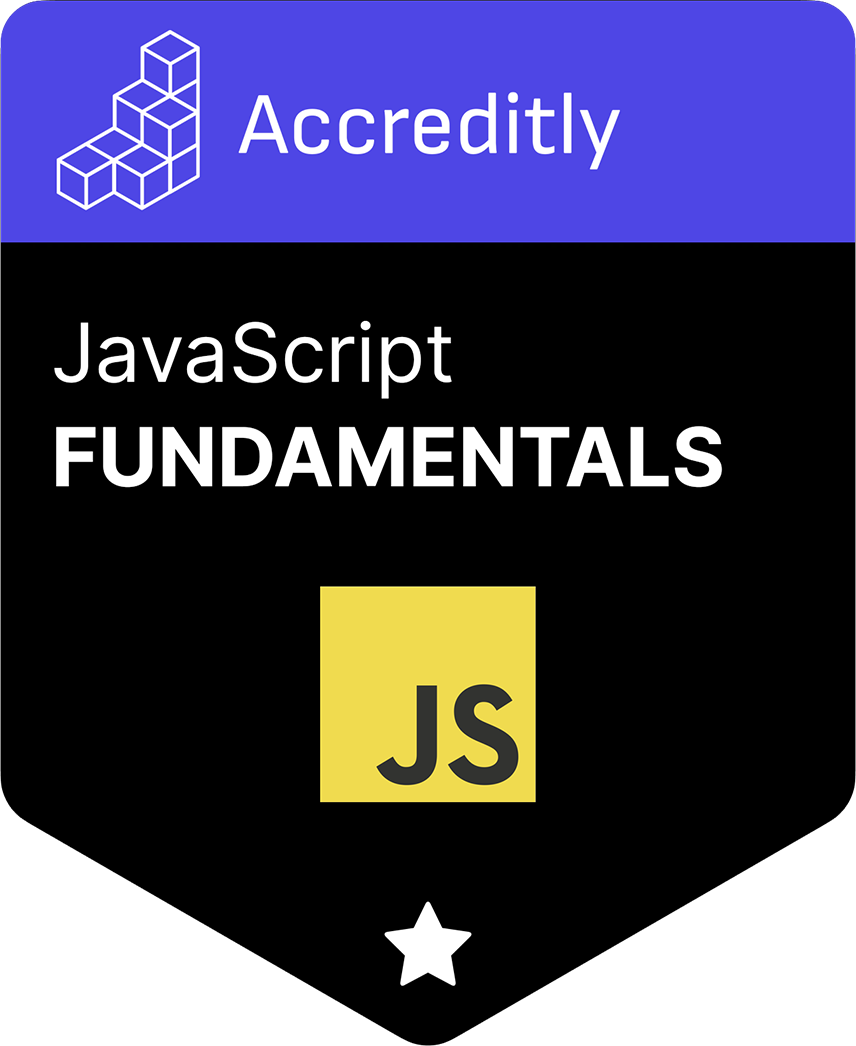