- Setting Up Your Environment
- Step 1: Create a Discord Application
- Step 2: Get Your Bot Token
- Step 3: Install a PHP Library
- Writing Your Discord Bot
- Create the Bot Script
- Running Your Bot
- Deploying Your Bot
Discord has become a popular platform for community engagement across various groups, from gaming to professional networks. Creating a custom Discord bot can enhance the interaction within these communities. While JavaScript is commonly used for such tasks, PHP developers can also create efficient Discord bots using specific libraries. This article will guide you through the process of writing a Discord bot in PHP, covering setup, coding, and deployment.
Setting Up Your Environment
Before coding the bot, you need to set up a Discord application and get the necessary credentials.
Step 1: Create a Discord Application
- Log In to Discord Developer Portal: Go to Discord Developer Portal and log in with your Discord account.
- Create a New Application: Click on the “New Application” button, give your application a name, and create it.
- Set Up Bot Account: In your application settings, navigate to the “Bot” tab and click “Add Bot”. You can customize your bot’s username and avatar here.
Step 2: Get Your Bot Token
After setting up your bot, you need to copy the bot token, which will be used to authenticate your bot with the Discord API.
- Copy the Token: In the bot settings under the “Bot” tab, find the token and click “Copy”. Keep this token secret, as it allows control over your bot.
Step 3: Install a PHP Library
To interact with the Discord API, you will use a PHP library that facilitates bot creation. One popular library for this purpose is discord-php/http
.
- Install via Composer: Run the following command in your project directory:
composer require team-reflex/discord-php
Writing Your Discord Bot
With the setup complete, you can now start coding your bot. Here is a simple example of how to connect your bot to Discord and make it respond to messages.
Create the Bot Script
Create a new PHP file called bot.php
and write the following code:
<?php
include 'vendor/autoload.php';
use Discord\Discord;
$discord = new Discord([
'token' => 'YOUR_BOT_TOKEN', // Replace 'YOUR_BOT_TOKEN' with your actual bot token
]);
$discord->on('ready', function ($discord) {
echo "Bot is ready!", PHP_EOL;
// Listen for messages
$discord->on('message', function ($message) {
echo "Received a message from {$message->author->username}: {$message->content}", PHP_EOL;
// Respond to a specific command
if ($message->content === '!hello') {
$message->channel->sendMessage('Hello, Discord!');
}
});
});
$discord->run();
This script uses the discord-php
library to create a connection to Discord using your bot token. It listens for messages and responds with "Hello, Discord!" whenever someone types !hello
in a channel your bot has access to.
Running Your Bot
To run your bot, simply execute the PHP script from the command line:
php bot.php
Ensure your PHP environment is configured to run scripts continuously and that you have an internet connection.
Deploying Your Bot
For your bot to run 24/7, you need to deploy it to a server. You can use any server that supports PHP and has a command line:
- Upload your bot files to the server.
- Install PHP and Composer if not already installed.
-
Run Composer to install dependencies:
composer install
. -
Use a process manager like
Supervisor
to keep your bot running continuously.
Creating a Discord bot in PHP is a straightforward process once you set up your environment and get familiar with the Discord API. PHP, with its vast ecosystem, provides robust solutions for developing complex bots capable of handling extensive interactions within Discord communities. Whether for fun, community management, or professional services, your PHP-based Discord bot can significantly enhance online interactions.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
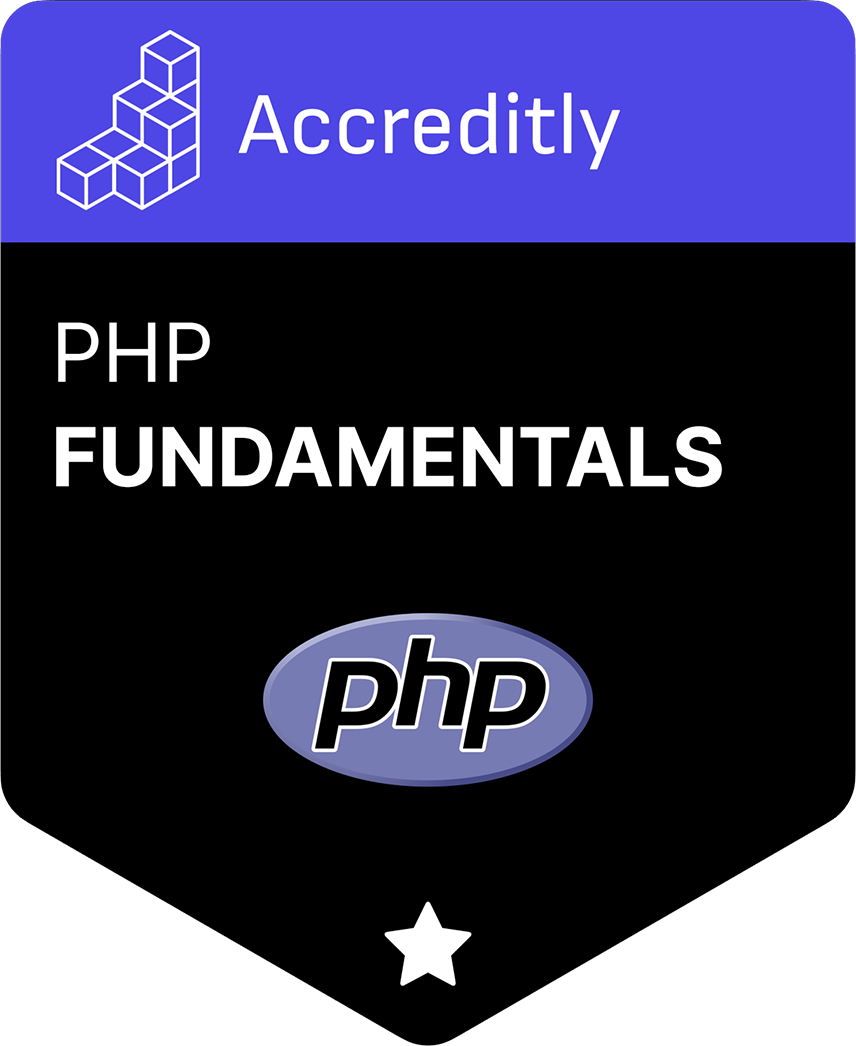