- Section 1: Understanding Exceptions and Errors in JavaScript
- Section 2: The try-catch Statement
- Section 3: Error Events
- Section 4: Error Handling with Promises and async/await
- Section 5: Custom Error Handling
- Summary
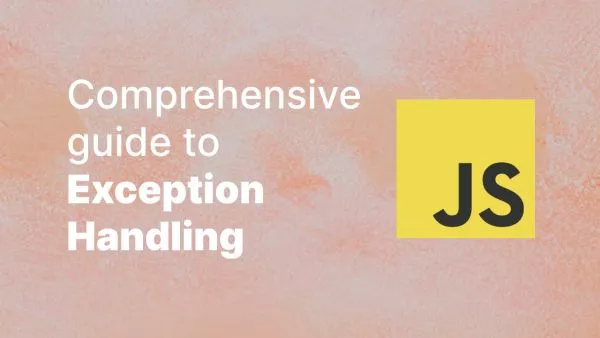
Exception handling is a crucial aspect of writing robust and maintainable JavaScript code. It allows developers to manage errors gracefully, ensuring that the application continues to function, even when unexpected issues arise. In this comprehensive guide, we will explore the different techniques for handling exceptions in JavaScript, including error types, try-catch blocks, error events, promises, and more.
If this all sounds like second nature to you then you might be interested in our JavaScript Fundamentals Certification, which covers best practices, security and error handling in JavaScript.
Section 1: Understanding Exceptions and Errors in JavaScript
1.1 Error Objects:
In JavaScript, errors are represented by the Error
object or one of its subclasses. The Error
object is a built-in object that provides a standard set of properties and methods for working with errors. The most common properties are name
and message
, which describe the error's type and a brief explanation, respectively.
Here's an example of creating a custom error object:
const customError = new Error('Something went wrong');
console.log(customError.name); // "Error"
console.log(customError.message); // "Something went wrong"
1.2 Built-in Error Types:
JavaScript provides several built-in error types that extend the base Error
object:
-
EvalError
: Raised when errors occur while evaluating code with theeval()
function. -
RangeError
: Occurs when a value is outside the allowable range, such as exceeding the maximum array length. -
ReferenceError
: Raised when trying to access a variable that doesn't exist or is out of scope. -
SyntaxError
: Occurs when a syntax error is detected in the code. -
TypeError
: Raised when an operation is performed on an incorrect data type, such as invoking a non-function value. -
URIError
: Occurs when incorrect usage of URI handling functions likeencodeURI()
ordecodeURI()
is detected.
const exampleRangeError = new RangeError('Invalid array length');
console.log(exampleRangeError.name); // "RangeError"
console.log(exampleRangeError.message); // "Invalid array length"
Section 2: The try-catch Statement
2.1 Basic Syntax:
The try-catch
statement is a fundamental exception handling mechanism in JavaScript. It allows you to execute a block of code and catch any exceptions that may occur during its execution. The basic syntax for a try-catch
statement is:
try {
// Code that might throw an exception
} catch (error) {
// Handle the exception
}
If an exception is thrown within the try
block, the code in the catch
block will be executed. The error parameter contains information about the exception.
2.2 Example of try-catch Usage:
function divide(a, b) {
if (b === 0) {
throw new Error('Division by zero is not allowed');
}
return a / b;
}
try {
const result = divide(10, 0);
console.log(result);
} catch (error) {
console.log(`An error occurred: ${error.message}`);
}
In this example, the divide()
function throws an error when attempting to divide by zero. The try-catch
block catches the error and logs the error message.
2.3 The finally Block:
The finally
block is an optional part of the try-catch
statement that is always executed, regardless of whether an exception was thrown or caught. It is useful for performing cleanup tasks, such as closing resources or releasing memory.
try {
// Code that might throw an exception
} catch (error) {
// Handle the exception
} finally {
// Code to always execute, regardless of whether an exception occurred
}
Here's an example using the finally
block:
let isOpen = false;
function openResource() {
isOpen = true;
console.log('Resource opened');
}
function closeResource() {
isOpen = false;
console.log('Resource closed');
}
try {
openResource();
// Code that might throw an exception
} catch (error) {
// Handle the exception
} finally {
closeResource();
}
In this example, the openResource()
function is called before the try
block, and the closeResource()
function is called within the finally
block. Regardless of whether an exception occurs, the finally
block ensures that the resource is closed.
Section 3: Error Events
3.1 Global Error Handlers:
When an error is not caught within a try-catch
block, it propagates to the global level, triggering the error event. You can attach an event listener to the window
object to handle global errors:
window.addEventListener('error', (event) => {
console.log(`An unhandled error occurred: ${event.message}`);
});
This technique is useful for logging unhandled errors or notifying users about issues.
3.2 Unhandled Promise Rejections:
Unhandled promise rejections trigger the unhandledrejection
event. You can listen for this event to handle unhandled promise rejections:
window.addEventListener('unhandledrejection', (event) => {
console.log(`An unhandled promise rejection occurred: ${event.reason}`);
});
Section 4: Error Handling with Promises and async/await
4.1 Catching Errors with Promises:
Promises provide a convenient way to handle asynchronous operations and errors. The catch()
method allows you to handle errors within a promise chain:
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.log(`An error occurred: ${error.message}`));
In this example, any errors that occur during the fetch()
operation or the subsequent then()
calls will be caught and handled by the catch()
method.
4.2 Handling Errors with async/await:
The async
/await
syntax allows you to handle errors in a more synchronous-like manner. You can use try-catch
blocks within an async
function to handle errors:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.log(`An error occurred: ${error.message}`);
}
}
fetchData();
In this example, the fetchData()
function is declared as async
, allowing the use of await
for asynchronous operations. If any errors occur, the try-catch
block will catch and handle them.
Section 5: Custom Error Handling
5.1 Creating Custom Error Classes:
To create more specific error types, you can extend the built-in Error
class:
class CustomError extends Error {
constructor(message) {
super(message);
this.name = 'CustomError';
}
}
try {
throw new CustomError('This is a custom error');
} catch (error) {
console.log(`${error.name}: ${error.message}`);
}
In this example, we define a CustomError
class that inherits from the built-in Error
class. This allows us to create custom error types with their own names and messages, making it easier to handle specific error cases.
5.2 Using Custom Error Classes in Practice:
Custom error classes can be used to handle specific error scenarios in your application more effectively. For example, you might create custom error classes for different types of validation errors:
class ValidationError extends Error {
constructor(message) {
super(message);
this.name = 'ValidationError';
}
}
class EmailValidationError extends ValidationError {
constructor(message) {
super(message);
this.name = 'EmailValidationError';
}
}
class PasswordValidationError extends ValidationError {
constructor(message) {
super(message);
this.name = 'PasswordValidationError';
}
}
function validateUserInput(email, password) {
if (!email.includes('@')) {
throw new EmailValidationError('Invalid email format');
}
if (password.length < 8) {
throw new PasswordValidationError('Password must be at least 8 characters long');
}
return true;
}
try {
validateUserInput('invalidemail.com', 'short');
} catch (error) {
if (error instanceof ValidationError) {
console.log(`Validation error: ${error.message}`);
} else {
console.log(`Unknown error: ${error.message}`);
}
}
In this example, we define custom error classes for different types of validation errors. The validateUserInput()
function throws specific errors based on the input. The try-catch
block handles these errors and logs the appropriate messages.
Summary
Exception handling is an essential part of writing robust and maintainable JavaScript code. By understanding the different techniques available, such as error objects, try-catch
blocks, error events, promises, async
/await
, and custom error handling, you can improve the resilience of your applications and handle unexpected issues gracefully.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
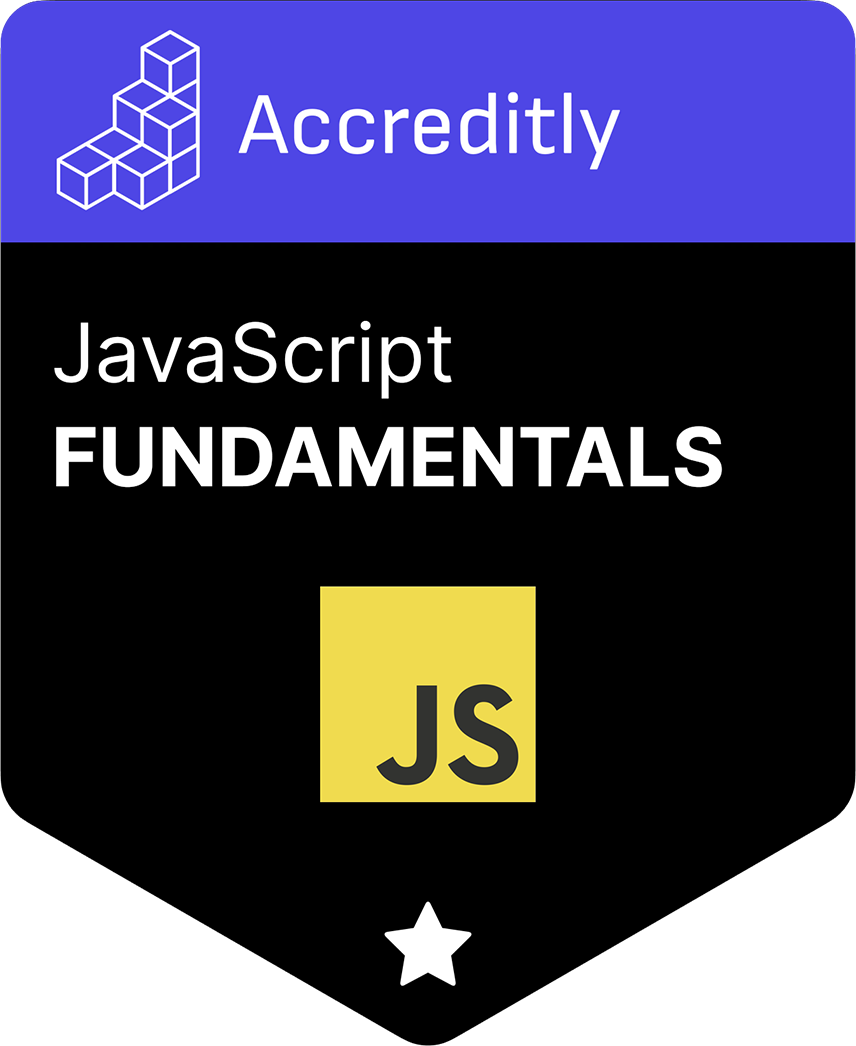