- Understanding event.key over keyCode
- Common Key Values and Their keyCode
- Detecting Key Actions
- Why Move Away from keyCode
- Conclusion
Creating a comprehensive and up-to-date list of all possible key values and their corresponding keycodes in JavaScript presents a challenge due to modern browser standards moving away from keyCode
properties in favor of event.key
. The use of keyCode
is deprecated, as it does not provide a reliable cross-platform or cross-browser way of identifying key actions, especially considering internationalization and accessibility. The event.key
property is now recommended for identifying keys because it is more readable and less dependent on the physical keyboard layout.
Below is a reference of some commonly used key values alongside their traditional keyCode
values, keeping in mind that you should prioritize using event.key
in new projects.
event.key
over keyCode
Understanding -
event.key
provides the value of the key pressed, making it easier to understand and maintain code. For example, pressing the A key will yield"a"
or"A"
depending on whether the shift key is also pressed. -
keyCode
, a numerical value, is deprecated and may not be supported in future web standards. It offered less readability and was more challenging to use without referencing a list of codes.
keyCode
Common Key Values and Their Below is a list combining both event.key
values for modern development practices and their corresponding (but deprecated) keyCode
values for reference:
Alphanumeric Keys
- A-Z:
event.key
is"a"
to"z"
(lowercase without shift key, uppercase with shift key),keyCode
is65
to90
- 0-9:
event.key
is"0"
to"9"
,keyCode
is48
to57
for top row numbers
Special Characters (vary by keyboard layout)
- Space:
event.key
is" "
,keyCode
is32
- Enter:
event.key
is"Enter"
,keyCode
is13
- Escape:
event.key
is"Escape"
,keyCode
is27
Control Keys
- Shift:
event.key
is"Shift"
,keyCode
is16
- Control (
Ctrl
):event.key
is"Control"
,keyCode
is17
- Alt:
event.key
is"Alt"
,keyCode
is18
- Backspace:
event.key
is"Backspace"
,keyCode
is8
- Tab:
event.key
is"Tab"
,keyCode
is9
Arrow Keys
- Left:
event.key
is"ArrowLeft"
,keyCode
is37
- Up:
event.key
is"ArrowUp"
,keyCode
is38
- Right:
event.key
is"ArrowRight"
,keyCode
is39
- Down:
event.key
is"ArrowDown"
,keyCode
is40
Function Keys
- F1-F12:
event.key
is"F1"
to"F12"
,keyCode
is112
to123
Detecting Key Actions
When detecting key presses, rely on event.key
:
document.addEventListener('keydown', (event) => {
console.log(`Key pressed: ${event.key}`);
});
keyCode
Why Move Away from The movement away from keyCode
towards event.key
was motivated by several factors:
-
Readability:
event.key
is much more understandable without referring to documentation. -
Internationalization:
event.key
is less dependent on the physical location of keys, making it more suitable for international applications. -
Deprecation and Future Support: Modern web standards recommend against using
keyCode
, as it may not be supported in future browsers and devices.
Conclusion
While historical keyCode
values are part of JavaScript's legacy in handling keyboard events, modern web development practices encourage the use of event.key
for a more readable, reliable, and future-proof approach. Transitioning to event.key
not only aligns with current web standards but also enhances the accessibility and internationalization of web projects. Remember, the key to successful keyboard event handling is understanding user needs and browser compatibility to create inclusive and functional web applications.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
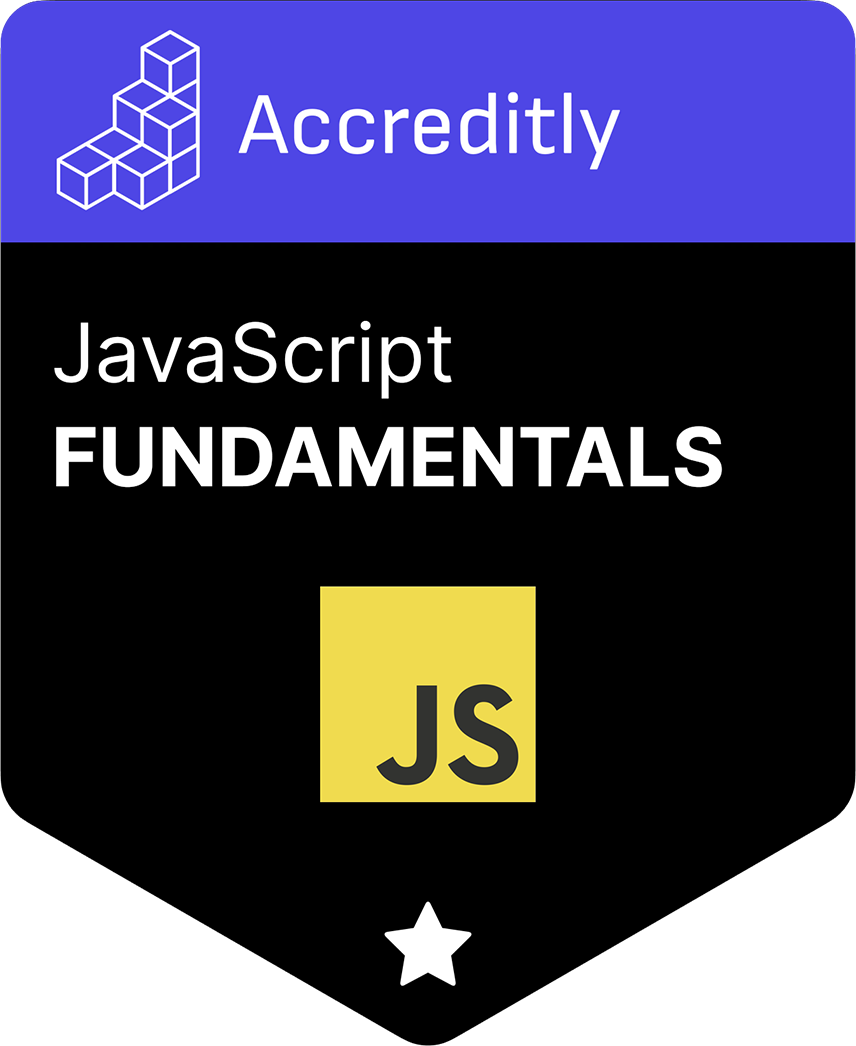
Related articles
Tutorials PHP Database Design Tooling
When and how to squash migrations
Learn about squashing migrations in Laravel, a pivotal technique for optimizing your application's efficiency and maintainability. This guide covers the why behind migration squashing and provides a tutorial on implementing it.