- Understanding Rate Limiting in Laravel
- Configuring Rate Limiting
- Creating Advanced Rate Limiting Strategies
- Testing and Monitoring
Rate limiting is a crucial aspect of web application security and integrity, safeguarding your app against overuse or abuse, such as brute-force attacks. Laravel includes robust support for rate limiting out of the box. This capability allows you to control how often a user can access certain functionality within a set period, thereby preventing server overload and ensuring equitable resource distribution. This article explores how to effectively implement a rate limiter in Laravel, ensuring your application remains performant and secure.
Understanding Rate Limiting in Laravel
Laravel's rate limiting features are built on top of Laravel's caching system and are primarily configured in the App\Http\Kernel.php
file or directly within specific routes. Laravel leverages the ThrottleRequests
middleware for this purpose, providing a flexible way to limit the number of requests to your application over a given time span.
Configuring Rate Limiting
Global Rate Limits with Middleware
-
Define the Rate Limiter: First, define your rate limiter in the
boot
method of yourApp\Providers\RouteServiceProvider
class. Laravel 8 introduced a rate limiter configuration method that supports defining complex rate limiting logic.
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
/**
* Configure the rate limiters for the application.
*/
protected function configureRateLimiting()
{
RateLimiter::for('global', function (Request $request) {
return Limit::perMinute(60)->by($request->ip());
});
}
In this example, a rate limiter named global
is defined, allowing 60 requests per minute per IP address.
-
Assign the Limiter to Routes: Next, assign the rate limiter to your routes using the
middleware
method. InApp\Http\Kernel.php
, make sure theThrottleRequests
middleware is registered, then apply it globally or to specific routes/groups.
protected $middlewareGroups = [
'web' => [
// Other middleware
'throttle:global', // Using the named rate limiter
],
'api' => [
// Other middleware
'throttle:api', // Laravel includes an 'api' rate limiter configuration by default
],
];
Custom Rate Limits for Specific Routes
You can also define custom rate limits directly on individual routes or route groups if you need more granular control:
use Illuminate\Support\Facades\Route;
Route::middleware('throttle:10,1')->group(function () {
Route::get('/rate-limited', function () {
// This route is limited to 10 requests per minute
});
});
This route definition limits access to 10 requests per minute.
Creating Advanced Rate Limiting Strategies
Laravel's rate limiting system is highly extensible, allowing you to implement more complex strategies, such as varying limits based on user authentication, roles, or other factors:
RateLimiter::for('dynamic', function (Request $request) {
if ($request->user()->isPremiumMember()) {
return Limit::perMinute(100);
}
return Limit::perMinute(10);
});
This dynamic rate limiter adjusts the request limit based on whether the user is marked as a premium member.
Testing and Monitoring
After implementing rate limiting, it's crucial to test your application under various conditions to ensure the rate limiter behaves as expected. Use tools like Laravel Telescope for monitoring and debugging rate limit hits and adjustments.
Implementing rate limiting in Laravel applications is a straightforward yet powerful way to enhance security and ensure your app's stability. By leveraging Laravel's built-in rate limiting capabilities, you can easily protect your application from excessive use and maintain a high level of service for all users. Whether applying global limits or crafting complex, conditional strategies, Laravel's flexible rate limiting features have you covered.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
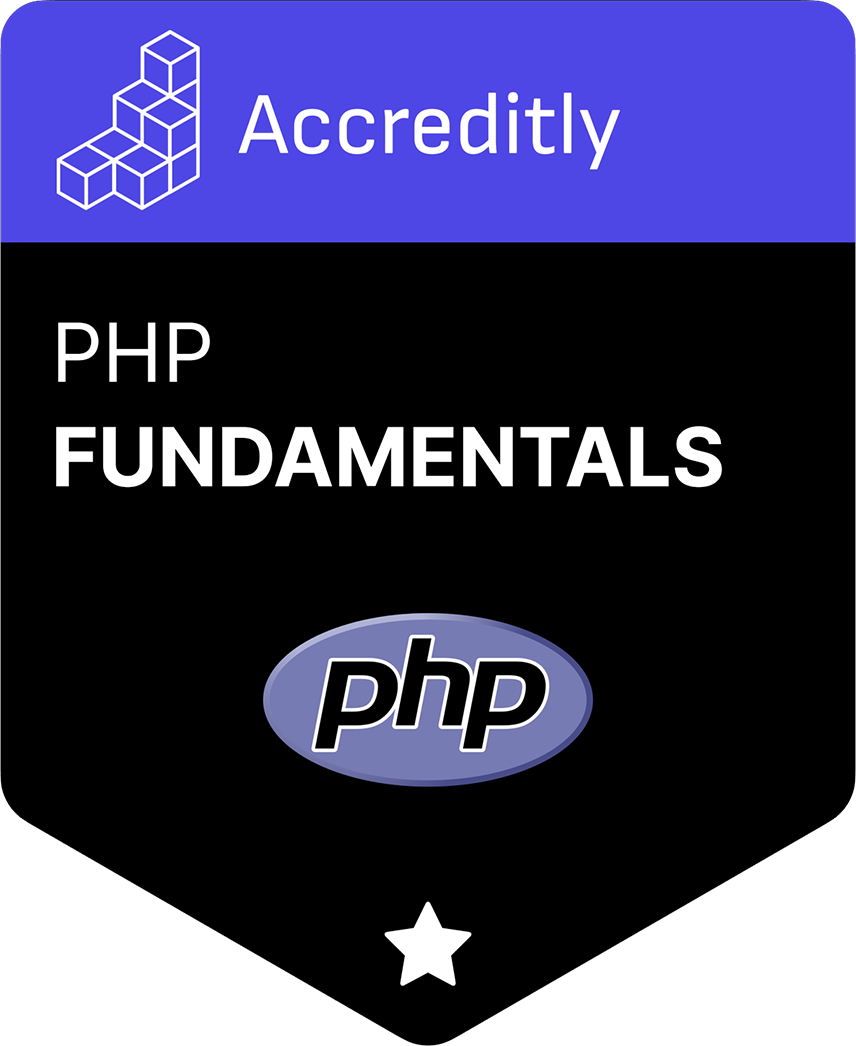