- Prerequisites
- Step 1: Create a Registration Form
- Step 2: Add the Page Template to a New Page
- Step 3: Validate Form Data
- Step 4: Create New User
WordPress comes with a built-in user registration form. However, it's rather basic and may not meet your needs. In this tutorial, we'll walk you through how to create a custom user registration form in the frontend, without using any plugin.
This tutorial is one of a number of tutorials we are creating in order to build your own frontend authentication in WordPress. To date we have discussed how to create a frontend login form on WordPress.
Prerequisites
- Basic knowledge of PHP and HTML.
- An active WordPress website where you can add custom code.
Step 1: Create a Registration Form
The first step is to create a custom page template that will display our registration form. In your active theme's directory, create a new PHP file custom-register.php
.
Paste the following code into custom-register.php
:
<?php
/*
Template Name: Custom Register
*/
// If the form is submitted
if($_SERVER['REQUEST_METHOD'] == 'POST') {
// We'll process the form in the next steps
}
get_header();
?>
<form method="post" action="<?php echo $_SERVER['REQUEST_URI']; ?>">
<div>
<label for="username">Username</label>
<input type="text" name="username" id="username">
</div>
<div>
<label for="password">Password</label>
<input type="password" name="password" id="password">
</div>
<div>
<label for="email">Email</label>
<input type="email" name="email" id="email">
</div>
<input type="submit" name="submit" value="Register">
</form>
<?php get_footer(); ?>
This is a basic form with fields for the username, password, and email. You can add more fields as needed.
Step 2: Add the Page Template to a New Page
In the WordPress admin, create a new page, and in the Page Attributes meta box, set the template to 'Custom Register'. Publish the page and view it to see your form.
Step 3: Validate Form Data
Now, we need to validate the form data when it's submitted. Inside the if
condition where we check for a POST request, add the following:
// Check username is present and doesn't already exist
$username = sanitize_user( $_POST['username'] );
if ( ! $username || username_exists( $username ) ) {
echo 'Please enter a valid username';
return;
}
// Check email address is present and valid
$email = sanitize_email( $_POST['email'] );
if ( ! $email || ! is_email( $email ) ) {
echo 'Please enter a valid email address';
return;
}
// Check password is valid
$password = $_POST['password'];
if ( ! $password ) {
echo 'Please enter a password';
return;
}
Step 4: Create New User
If the form data is valid, we can create a new user with wp_create_user()
. Add the following at the end of the if
condition:
// All validation checks passed successfully. Proceed to create the user.
$user_id = wp_create_user( $username, $password, $email );
if ( ! is_wp_error( $user_id ) ) {
echo 'Your registration was successful.';
} else {
echo 'There was an error in the registration process.';
}
In this tutorial, you've created a custom registration form that validates user input and creates a new user. This is a basic form but serves as a great starting point for more complex registration forms.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
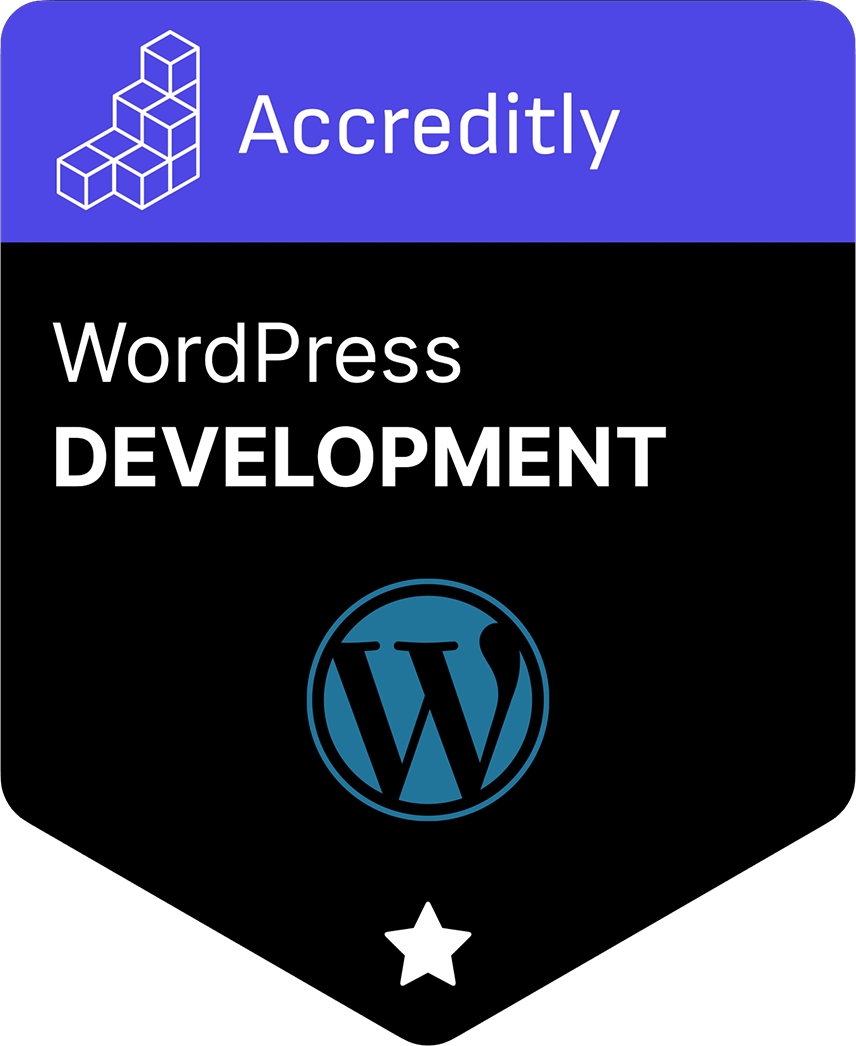