- Step 1: Create the Plugin
- Step 2: Create a Custom Admin Page
- Step 3: Display the Map on the Frontend
Before we begin we'll be using Google Maps API, so you'll need to ensure you have a working key which is available from the Google Maps API site. Google Maps keys are published in the source code of the website, so they're readable by your visitors. As such, make sure your key is locked down to your website to stop it being used by anyone.
Step 1: Create the Plugin
Start by creating a new directory inside your wp-content/plugins
folder. Let's call it address-map
. Inside this directory, create a new file called address-map.php
. This will be the main file for your plugin.
In this file, start with a plugin header comment, which WordPress uses to display information about the plugin in the Dashboard:
<?php
/**
* Plugin Name: Address Map
* Description: A simple plugin that adds an address input in the admin and plots a point on a map on the frontend.
* Version: 1.0
* Author: Your Name
* Author URI: https://accreditly.io
*/
Step 2: Create a Custom Admin Page
Next, let's add a custom admin page to WordPress where we can input an address. We'll use the admin_menu
action hook to add our custom page. We'll also enqueue a script file for our admin page:
function address_map_admin_menu() {
add_menu_page(
'Address Map Settings',
'Address Map',
'manage_options',
'address-map',
'address_map_admin_page',
'dashicons-location',
20
);
}
function address_map_admin_page() {
?>
<div class="wrap">
<h1>Address Map Settings</h1>
<form method="post" action="options.php">
<?php
settings_fields('address_map_options');
do_settings_sections('address_map');
submit_button();
?>
</form>
</div>
<?php
}
function address_map_settings() {
add_settings_section('address_map_main', 'Main Settings', null, 'address_map');
add_settings_field('address_map_address', 'Address', 'address_map_address_input', 'address_map', 'address_map_main');
register_setting('address_map_options', 'address_map_address');
}
function address_map_address_input() {
$address = get_option('address_map_address');
echo "<input id='address_map_address' name='address_map_address' type='text' value='" . esc_attr($address) . "'>";
}
add_action('admin_menu', 'address_map_admin_menu');
add_action('admin_init', 'address_map_settings');
In this code, we first add a new menu page using add_menu_page(). We then define the contents of the page in the address_map_admin_page()
function, where we create a form with settings fields.
The address_map_settings()
function is where we add our settings section and field, and register the option address_map_address
.
The address_map_address_input()
function outputs an input field for our address.
Step 3: Display the Map on the Frontend
We'll use the Google Maps JavaScript API to display a map on the frontend and plot a point based on the address input in the admin.
First, enqueue the Google Maps script in your functions.php
file, replacing your-api-key
with your actual API key:
function address_map_scripts() {
wp_enqueue_script('google-maps', 'https://maps.googleapis.com/maps/api/js?key=your-api-key', array(), null, true);
}
add_action('wp_enqueue_scripts', 'address_map_scripts');
Next, create a short code to output the map:
function address_map_shortcode($atts) {
$address = get_option('address_map_address');
ob_start();
?>
<div id="map" style="height: 400px; width: 100%;"></div>
<script>
var geocoder;
var map;
function initMap() {
geocoder = new google.maps.Geocoder();
var latlng = new google.maps.LatLng(-34.397, 150.644);
var mapOptions = {
zoom: 8,
center: latlng
}
map = new google.maps.Map(document.getElementById('map'), mapOptions);
geocodeAddress(geocoder, map);
}
function geocodeAddress(geocoder, resultsMap) {
var address = '<?php echo esc_js($address); ?>';
geocoder.geocode({'address': address}, function(results, status) {
if (status == 'OK') {
resultsMap.setCenter(results[0].geometry.location);
var marker = new google.maps.Marker({
map: resultsMap,
position: results[0].geometry.location
});
} else {
alert('Geocode was not successful for the following reason: ' + status);
}
});
}
google.maps.event.addDomListener(window, 'load', initMap);
</script>
<?php
return ob_get_clean();
}
add_shortcode('address_map', 'address_map_shortcode');
In this code, we first get the address that was input in the admin. We then output a div to contain our map, and a script to handle the map functionality. The script creates a map and a geocoder, which is used to convert the address to a latitude/longitude coordinate that the map can use.
The short code can now be used in any post or page to display the map: [address_map]
That's it! In this basic example the map will display, and will fire an alert to the browser if there are issues. It is a very basic example and can be easily extended to include extra features, such as:
- A typeahead field in the admin to pre-select the address
- Customisations to the map visuals
- Different map types
- Better error handling
- Adding customisable content on the pin on the map
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
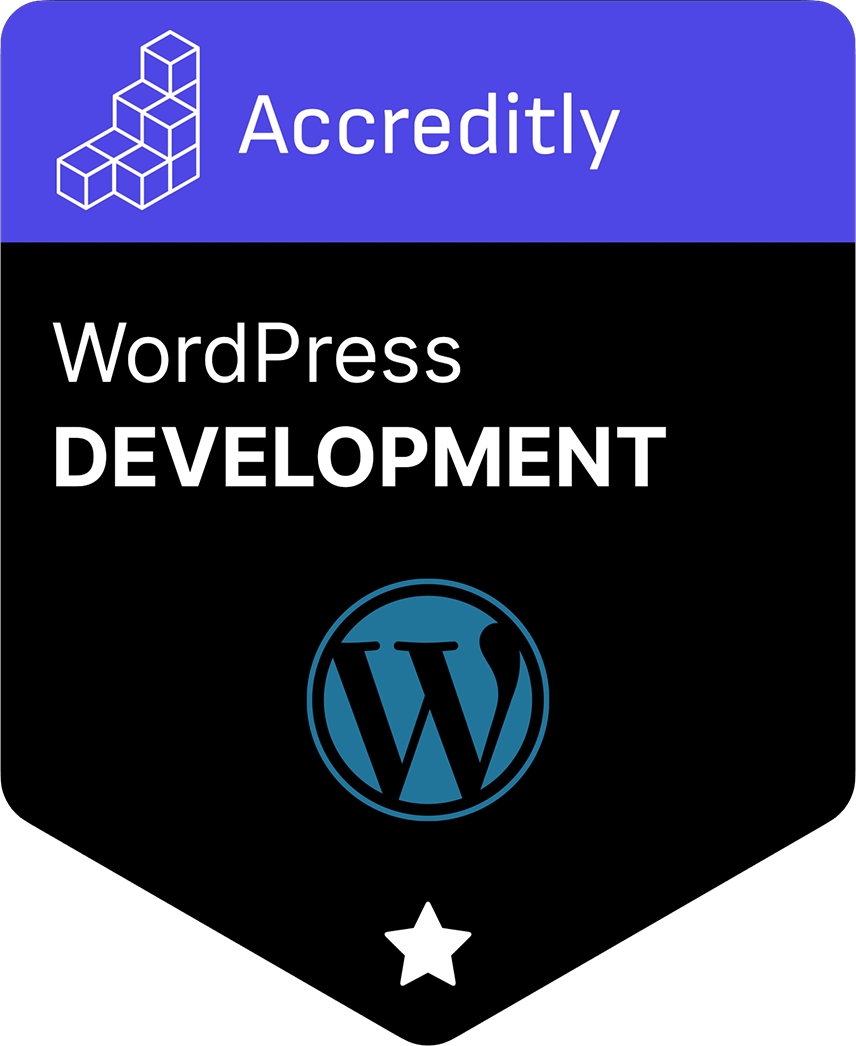