- Understanding the WordPress Admin Area
- Customizing the Admin Menu
- Customizing the Admin Bar
- Customizing Dashboard Widgets
- Customizing the Login Page
- Customizing the Admin Footer
- Tailoring the WordPress Admin Area for Optimal Use
As a WordPress developer, customizing the admin area can significantly enhance the user experience for your clients. While plugins offer quick solutions, they can bloat your site and may not always meet specific needs. Customizing the admin area through code provides a streamlined, efficient, and tailored experience. This article delves into various methods to customize the WordPress admin area without relying on plugins, ensuring you have full control over the functionality and aesthetics.
Understanding the WordPress Admin Area
Before diving into customization, it’s essential to understand the components of the WordPress admin area. The admin area, or the dashboard, is where users manage their content, settings, and plugins. It comprises the admin menu, admin bar, dashboard widgets, and other settings pages.
Customizing the Admin Menu
The admin menu is the primary navigation tool in the WordPress dashboard. Customizing it can help streamline the workflow by removing unnecessary items, renaming menus, or adding new ones. Here’s how you can achieve that with code.
Removing Menu Items
To remove menu items, use the remove_menu_page()
function. Add the following code to your theme’s functions.php
file or a custom plugin:
function remove_menus(){
remove_menu_page('edit.php'); // Posts
remove_menu_page('upload.php'); // Media
remove_menu_page('edit.php?post_type=page'); // Pages
remove_menu_page('edit-comments.php'); // Comments
// Add more as needed
}
add_action('admin_menu', 'remove_menus');
Renaming Menu Items
To rename menu items, use the global $menu
and global $submenu
arrays. Here’s an example:
function rename_admin_menu_items() {
global $menu;
global $submenu;
$menu[5][0] = 'Articles'; // Change 'Posts' to 'Articles'
// Add more as needed
}
add_action('admin_menu', 'rename_admin_menu_items');
Adding Custom Menu Items
To add custom menu items, use the add_menu_page()
function:
function add_custom_menu_item() {
add_menu_page(
'Custom Menu Title',
'Custom Menu',
'manage_options',
'custom-menu-slug',
'custom_menu_page_callback',
'dashicons-admin-generic',
6
);
}
function custom_menu_page_callback() {
echo '<h1>Custom Menu Page Content</h1>';
}
add_action('admin_menu', 'add_custom_menu_item');
Customizing the Admin Bar
The admin bar, located at the top of the dashboard, can also be customized to improve user experience. You can remove, rename, or add new items to the admin bar.
Removing Admin Bar Items
To remove items from the admin bar, use the remove_node()
method:
function customize_admin_bar($wp_admin_bar) {
$wp_admin_bar->remove_node('wp-logo'); // Remove WordPress logo
$wp_admin_bar->remove_node('comments'); // Remove comments link
// Add more as needed
}
add_action('admin_bar_menu', 'customize_admin_bar', 999);
Adding Custom Admin Bar Items
To add custom items to the admin bar, use the add_node()
method:
function add_custom_admin_bar_item($wp_admin_bar) {
$args = array(
'id' => 'custom-item',
'title' => 'Custom Item',
'href' => '/custom-url',
'meta' => array(
'class' => 'custom-item-class',
'title' => 'Custom Tooltip'
)
);
$wp_admin_bar->add_node($args);
}
add_action('admin_bar_menu', 'add_custom_admin_bar_item', 999);
Customizing Dashboard Widgets
Dashboard widgets provide a quick overview of the site’s status and activity. Customizing these widgets can enhance the dashboard’s functionality and relevance.
Removing Default Widgets
To remove default widgets, use the remove_meta_box()
function:
function remove_dashboard_widgets() {
remove_meta_box('dashboard_quick_press', 'dashboard', 'side'); // Quick Draft
remove_meta_box('dashboard_recent_drafts', 'dashboard', 'side'); // Recent Drafts
remove_meta_box('dashboard_primary', 'dashboard', 'side'); // WordPress News
remove_meta_box('dashboard_secondary', 'dashboard', 'side'); // Other WordPress News
// Add more as needed
}
add_action('wp_dashboard_setup', 'remove_dashboard_widgets');
Adding Custom Widgets
To add custom dashboard widgets, use the wp_add_dashboard_widget()
function:
function add_custom_dashboard_widget() {
wp_add_dashboard_widget(
'custom_dashboard_widget',
'Custom Widget Title',
'custom_dashboard_widget_content'
);
}
function custom_dashboard_widget_content() {
echo '<p>Custom widget content goes here.</p>';
}
add_action('wp_dashboard_setup', 'add_custom_dashboard_widget');
Customizing the Login Page
Customizing the login page can enhance branding and user experience. This can be achieved by altering the logo, background, and form styles.
Changing the Login Logo
To change the login logo, use the following code:
function custom_login_logo() {
echo '
<style type="text/css">
#login h1 a {
background-image: url(' . get_stylesheet_directory_uri() . '/images/custom-logo.png);
width: 300px;
background-size: contain;
}
</style>
';
}
add_action('login_enqueue_scripts', 'custom_login_logo');
Customizing the Login Styles
To customize the login styles, add the following code:
function custom_login_styles() {
echo '
<style type="text/css">
body.login {
background-color: #f1f1f1;
}
.login form {
box-shadow: 0 0 10px rgba(0,0,0,0.1);
}
</style>
';
}
add_action('login_enqueue_scripts', 'custom_login_styles');
Customizing the Admin Footer
The footer message in the admin area can be customized to display your custom text or links.
function custom_admin_footer() {
echo 'Customized by Your Name. Visit <a href="https://yourwebsite.com">Your Website</a>.';
}
add_filter('admin_footer_text', 'custom_admin_footer');
Tailoring the WordPress Admin Area for Optimal Use
Customizing the WordPress admin area through code offers developers the flexibility to create a streamlined, efficient, and user-friendly experience. By adjusting the admin menu, admin bar, dashboard widgets, login page, and footer, you can tailor the dashboard to meet the specific needs of your clients or projects. Embrace these customization techniques to enhance your WordPress sites, providing a professional and personalized touch that stands out.
For more detailed information on WordPress customization, refer to the WordPress Codex and the WordPress Developer Handbook.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
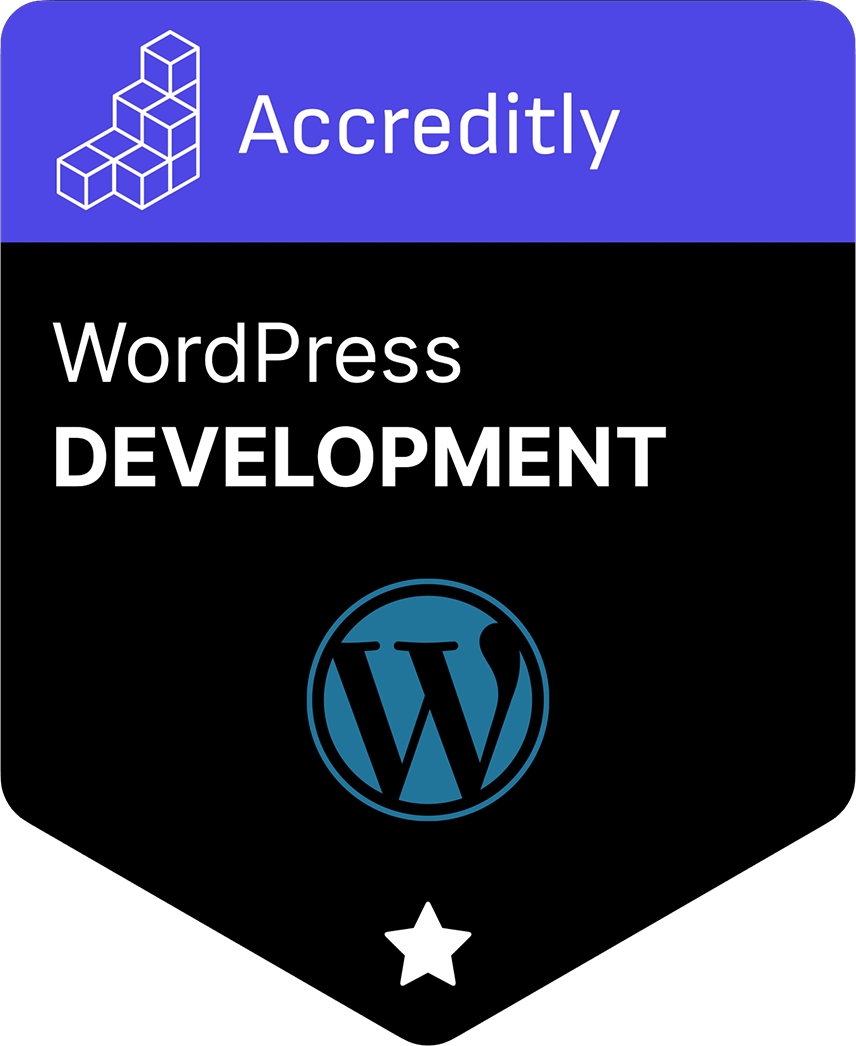