- 1. Accessing .env Variables in Node.js
- 2. Accessing .env Variables in Create React App
- 3. Caution for Frontend Apps
- 4. Accessing .env Variables in Other Bundlers
- 5. Best Practices
Environmental variables provide a way to keep your application scalable and secure, separating configuration from code. When working with JavaScript, you might encounter situations where you need to access environment variables, especially if you're on the server-side with Node.js or using frontend frameworks like React.
This guide explores how you can access .env file variables in different JavaScript contexts.
.env
Variables in Node.js
1. Accessing dotenv
Setting up To use .env
in Node.js, the popular dotenv package is often used.
Install the package:
npm install dotenv
In your main server file (e.g., index.js or server.js), add:
require('dotenv').config();
Using the Variables
Once set up, you can access any variables from your .env
file using process.env.YOUR_VARIABLE_NAME
:
const PORT = process.env.PORT || 3000;
2. Accessing .env Variables in Create React App
Create React App (CRA) has built-in support for .env
files, but with a catch.
Prefixing Variable Names
Only variables starting with REACT_APP_
are embedded into the build. For example:
REACT_APP_API_URL=https://api.example.com
Using the Variables
In your React components or any JavaScript files in the CRA project:
const apiUrl = process.env.REACT_APP_API_URL;
3. Caution for Frontend Apps
It's essential to understand that ANYTHING on the frontend is exposed to the client. This means all your environment variables bundled in your frontend code can be seen by viewing the page's source code. Never put sensitive information, like API keys, in client-side code.
.env
Variables in Other Bundlers
4. Accessing For bundlers like Webpack, Rollup, or Parcel, plugins or configurations allow you to replace environment variables during the bundling process. These methods usually involve reading .env
files and replacing placeholders in your code with the actual values during build time.
5. Best Practices
- Keep Secrets Secret: Never expose sensitive data like API keys, database credentials, etc., on the client-side.
- Use Fallbacks: Always have a default value in case an environment variable isn't set. This helps in avoiding unexpected behaviors.
- Version Control: Avoid pushing your
.env
file to version control. Use.gitignore
to exclude it. Instead, you can have a.env.example
file that outlines the required variables without the values.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
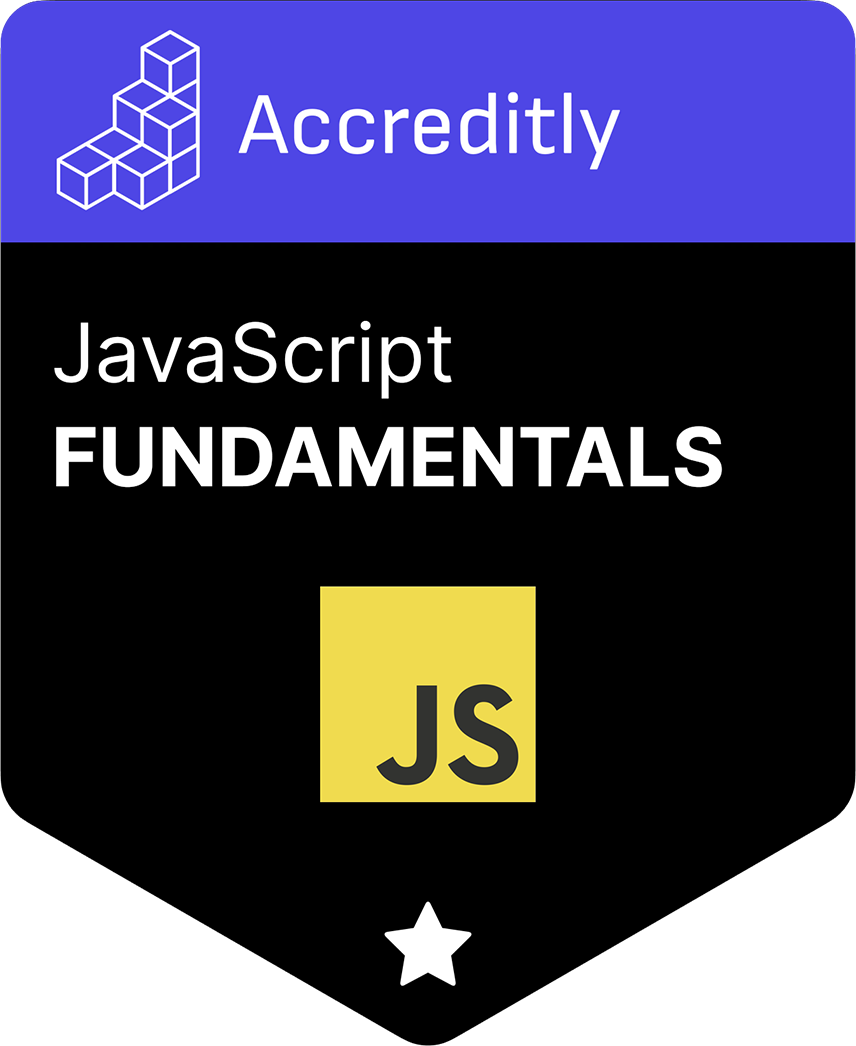