- Choosing a QR Code Generation Library
- Step 1: Install PHP QR Code Library
- Step 2: Generate a QR Code
- Step 3: Customize Your QR Code
- Step 4: Save the QR Code to a File
QR codes (Quick Response Codes) have become a ubiquitous tool for quick access to URLs, product information, and various other data types using smartphones. In PHP, generating QR codes can be highly useful for a range of applications, from marketing promotions to user authentication. This article will explain how to generate QR codes in PHP using popular libraries, ensuring you can integrate this functionality smoothly into your projects.
Choosing a QR Code Generation Library
Several PHP libraries can generate QR codes, each offering different features and customization options. The most popular among these are PHP QR Code and Google Chart API. For the purpose of this tutorial, we will focus on the PHP QR Code library due to its simplicity and ease of use without needing external API calls.
Step 1: Install PHP QR Code Library
The PHP QR Code library is an open-source library that can generate QR codes in PHP without any external dependencies. It can be installed manually or via Composer.
To install via Composer:
-
If you haven't already installed Composer, download and install it from getcomposer.org.
-
Run the following command in your project directory:
composer require chillerlan/php-qrcode
This command installs the chillerlan/php-qrcode
package, which provides a modern interface to generating QR codes compatible with PHP 7.2 and newer.
Step 2: Generate a QR Code
Once the library is installed, you can start generating QR codes. Here’s how to create a basic QR code:
<?php
require_once 'vendor/autoload.php';
use chillerlan\QRCode\QRCode;
use chillerlan\QRCode\QROptions;
// Set up QR code settings
$options = new QROptions([
'version' => 5,
'outputType' => QRCode::OUTPUT_IMAGE_PNG,
'eccLevel' => QRCode::ECC_L,
]);
// Create QR code data
$data = 'https://www.example.com'; // URL or text to encode into the QR code
// Instantiate QRCode object with options
$qrcode = new QRCode($options);
// Generate and output the QR code
header('Content-type: image/png');
echo $qrcode->render($data);
This script generates a QR code for the URL https://www.example.com
. The QR code's specifications, such as its version, error correction level, and output type, are customizable through the QROptions
class.
Step 3: Customize Your QR Code
The chillerlan/php-qrcode
library allows extensive customization of the QR code. You can modify the size, colors, logo embedding, and much more. Here’s an example of setting a few more options:
$options = new QROptions([
'version' => 7,
'outputType' => QRCode::OUTPUT_IMAGE_PNG,
'eccLevel' => QRCode::ECC_H,
'scale' => 5,
'imageTransparent' => false, // No transparency
'imageBase64' => false, // Output raw image data
]);
// Generate QR code with advanced options
echo $qrcode->render($data);
Step 4: Save the QR Code to a File
Instead of directly outputting the QR code to the browser, you might want to save it to a file:
$filepath = 'path/to/qrcode.png';
$qrcode->render($data, $filepath);
This will save the QR code as a PNG file in the specified path.
Generating QR codes in PHP is a straightforward process with the help of robust libraries like chillerlan/php-qrcode
. Whether embedding URLs for websites, encoding personal information, or creating QR codes for event tickets, the ability to generate QR codes dynamically enhances the functionality of your PHP applications. Always ensure that the data encoded in QR codes is secure and does not expose sensitive information inadvertently.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
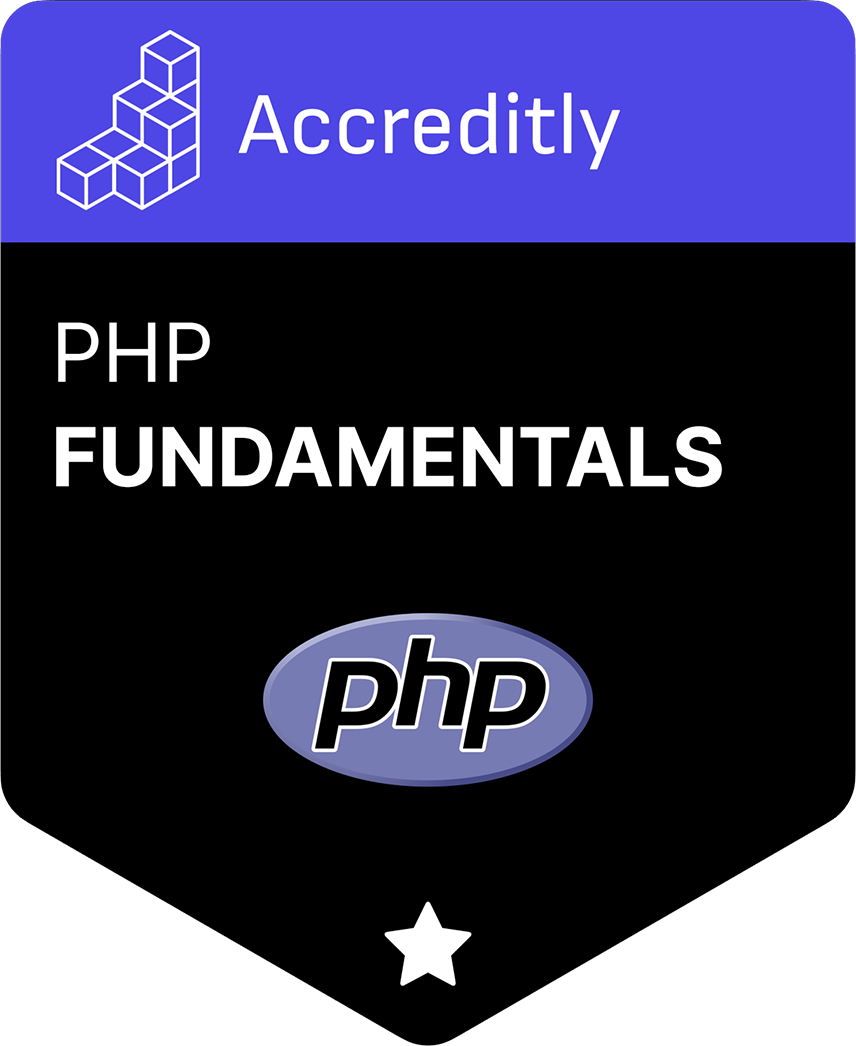