When dealing with very large files in PHP, traditional methods of file handling can often lead to memory exhaustion. This is because they typically read the entire file into memory at once. Instead, it's often better to read the file incrementally, processing each piece as it comes in. This allows us to keep memory usage down, even with extremely large files.
One way to accomplish this is by using PHP's generators. Generators provide an easy way to implement simple iterators without the complexity associated with classes that implement the Iterator interface.
What are PHP Generators?
Generators are a feature of PHP that allows you to write a function that behaves like an iterator. This means it can be used in a foreach
loop to iterate over a set of data without needing to build an array in memory. It does this by yielding values one at a time, only generating the next value when it's needed.
Parsing a Large File with Generators
Now let's see how we can use generators to parse a large file. We'll create a function that opens a file and yields one line at a time. This function will only read one line into memory at a time, which makes it suitable for large files.
Here's an example of a generator function that reads a file line by line:
function readLargeFile($fileName) {
$file = fopen($fileName, 'r');
if (!$file) throw new Exception('Could not open file');
while (($line = fgets($file)) !== false) {
yield $line;
}
fclose($file);
}
This function opens a file and starts a loop. On each iteration, it reads a single line from the file using fgets()
. It then yields that line, allowing it to be processed outside the function before the next line is read.
You can use this function in a foreach loop to process each line individually, like this:
foreach (readLargeFile('large-file.txt') as $line) {
// Process the line here
echo $line;
}
This will echo each line of the file individually, and because of the generator function, only one line is held in memory at a time. This method can be used to process very large files without consuming a lot of memory.
Remember, dealing with large files in PHP may still have its limitations depending on your server setup, and it's important to consider other factors like execution time and performance. Always ensure your solution is fit for your specific needs and thoroughly tested.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
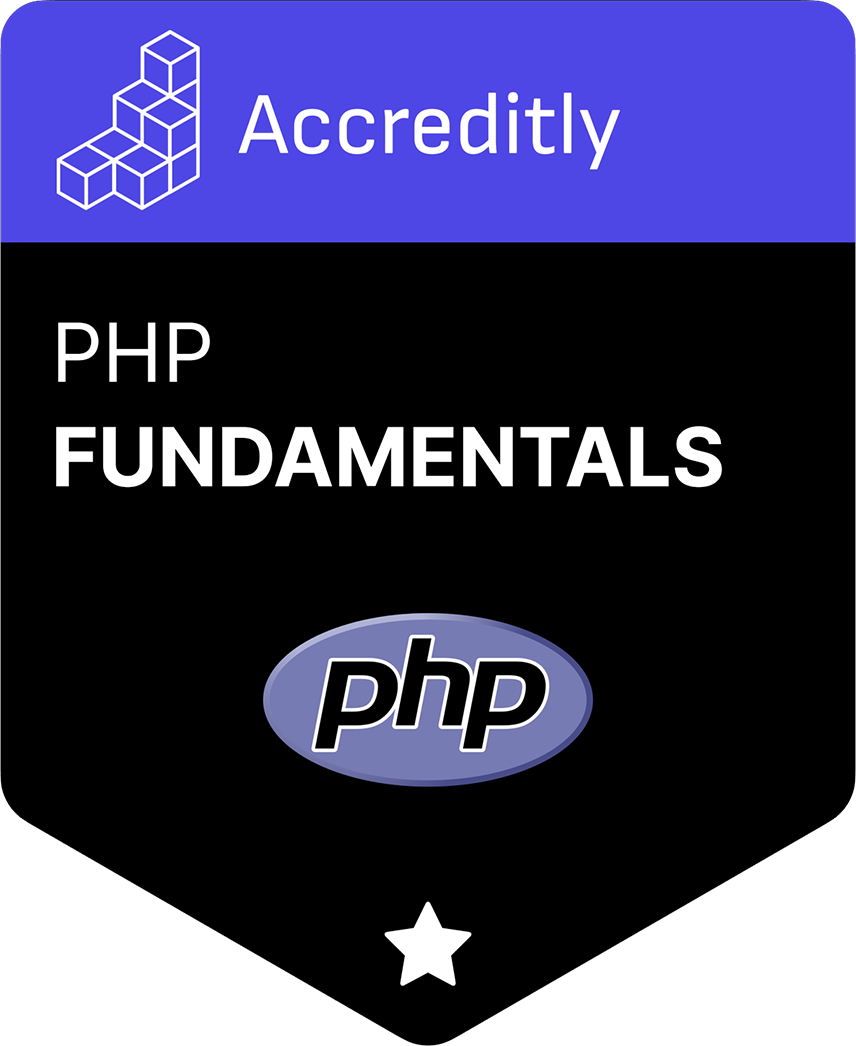