- 1. Selecting Elements by ID
- 2. Selecting Elements by Class Name
- 3. Selecting Elements by Tag Name
- 4. Using CSS Selectors
- 5. Selecting the First Matching Element
- 6. Traversing the DOM
- 7. Filtering Selection
- A Subtle Nudge Towards Native JavaScript
jQuery, once the go-to library for web developers, revolutionized how we approached JavaScript and the DOM. Its concise syntax and cross-browser compatibility made tasks like DOM selection a breeze. Fast forward to today, native JavaScript has evolved tremendously, offering similar, if not better, utilities for DOM manipulation without the need for external libraries.
There's a growing consensus among developers: If you can avoid jQuery and lean on modern native JavaScript, you should. The reasons are compelling: reduced page load times, better performance, and fewer dependencies to maintain. Let's compare popular jQuery selectors with their native JavaScript counterparts.
1. Selecting Elements by ID
jQuery:
$("#myId");
Native JavaScript:
document.getElementById("myId");
2. Selecting Elements by Class Name
jQuery:
$(".myClass");
Native JavaScript:
document.getElementsByClassName("myClass");
3. Selecting Elements by Tag Name
jQuery:
$("div");
Native JavaScript:
document.getElementsByTagName("div");
4. Using CSS Selectors
jQuery:
$("div.myClass > p");
Native JavaScript:
document.querySelectorAll("div.myClass > p");
5. Selecting the First Matching Element
jQuery:
$("p.myClass:first");
Native JavaScript:
document.querySelector("p.myClass");
6. Traversing the DOM
jQuery - Parent Element:
$("#myId").parent();
Native JavaScript:
document.getElementById("myId").parentNode;
jQuery - Child Elements:
$("#myId").children();
Native JavaScript:
document.getElementById("myId").children;
jQuery - Sibling Elements:
$("#myId").siblings();
Native JavaScript:
document.getElementById("myId").parentNode.children;
7. Filtering Selection
jQuery:
$("div").filter(".myClass");
Native JavaScript:
Array.from(document.querySelectorAll("div")).filter(el => el.classList.contains("myClass"));
A Subtle Nudge Towards Native JavaScript
Over the past few years, browsers have become remarkably advanced, rendering many of jQuery's features redundant. Native JavaScript now offers most of the conveniences for which we once turned to jQuery. Furthermore, by sidestepping jQuery, we sidestep an additional layer of abstraction, often leading to more transparent, maintainable, and performant code.
That said, jQuery still has its place, especially in legacy projects or specific scenarios where its unique features shine. However, for new projects or when optimizing existing ones, embracing vanilla JavaScript for DOM manipulation is a wise move.
As JavaScript continues to evolve, the gap between what jQuery offers and what native JavaScript can achieve narrows. Familiarising oneself with native methods and patterns can not only reduce external dependencies but also enhance the performance and maintainability of web applications. Happy coding without the jQuery crutch!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
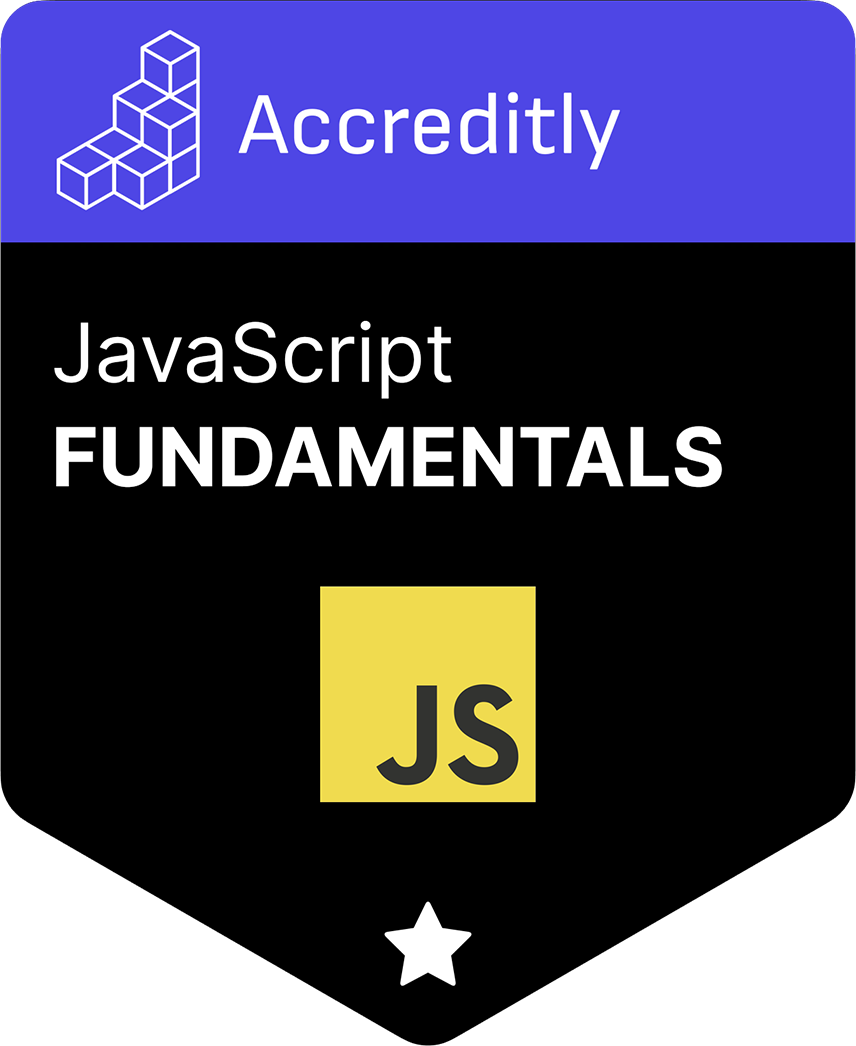