- Why Runtime Mailers?
- Using Laravel::Build for Runtime Mailers
- Real-World Example 1: User-Specific SMTP Settings
- Real-World Example 2: Switching Mail Providers Dynamically
- Real-World Example 3: Debugging and Testing
- Best Practices for Runtime Mailers
Laravel makes sending emails a breeze with its built-in Mail facade and the powerful mailer configurations it offers. But what happens when you need to dynamically change the email configuration during runtime? This might be necessary if you're building an application where users send emails via their own SMTP settings, or where you switch configurations based on the email type or recipient domain.
In this article, we'll explore how you can use the Mail facade in Laravel to configure runtime mailers using the Laravel::Build
feature. We'll also walk through real-world examples and show how this flexibility can make your application's email handling more dynamic and user-centric.
Why Runtime Mailers?
Most Laravel applications rely on a single static mail configuration set in the config/mail.php
file. While this works for many cases, certain scenarios call for more flexibility:
- User-specific SMTP Settings: For apps like CRMs or email marketing tools, users might want emails sent from their own email servers (Gmail, Office365, etc.).
- Dynamic Mail Providers: You might need to route some emails via different providers (e.g., SendGrid for transactional emails and Postmark for marketing emails).
- Debugging/Testing Environments: Sending emails through custom environments during testing or development stages.
To meet these demands, Laravel's Mail::build
functionality allows you to create mailers dynamically, giving you the power to adjust mailer configurations at runtime.
Laravel::Build
for Runtime Mailers
Using The Laravel::Build
feature lets you define mail configurations on the fly without having to alter your application's static configuration file. This approach creates a temporary mailer instance configured specifically for the email being sent.
Here's the syntax to dynamically configure and send an email:
use Illuminate\Support\Facades\Mail;
Mail::mailer('smtp')
->build(function ($mailer) {
$mailer->setHost('smtp.mailtrap.io');
$mailer->setPort(2525);
$mailer->setEncryption('tls');
$mailer->setUsername('your-smtp-username');
$mailer->setPassword('your-smtp-password');
})
->to('[email protected]')
->send(new YourMailable());
In the above code:
- The
build
method allows you to define specific configurations (like host, port, encryption, username, and password) for this mailer instance. - The email is sent via this temporary configuration without modifying the default settings in
config/mail.php
.
Real-World Example 1: User-Specific SMTP Settings
Imagine a CRM application where users want emails sent from their own SMTP accounts. For example, a user might have their own Gmail or Outlook SMTP credentials, and they expect emails to be sent using those credentials.
Implementation:
Here's how you can achieve this:
- Collect User SMTP Details: Store the user's SMTP details in your database when they configure their account.
Schema::create('smtp_settings', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained()->onDelete('cascade');
$table->string('host');
$table->integer('port');
$table->string('encryption');
$table->string('username');
$table->string('password');
$table->timestamps();
});
- Send Emails Using Dynamic SMTP Settings:
use Illuminate\Support\Facades\Mail;
use App\Models\SmtpSetting;
use App\Mail\UserNotification;
public function sendEmail($userId)
{
$smtp = SmtpSetting::where('user_id', $userId)->firstOrFail();
Mail::mailer('smtp')
->build(function ($mailer) use ($smtp) {
$mailer->setHost($smtp->host);
$mailer->setPort($smtp->port);
$mailer->setEncryption($smtp->encryption);
$mailer->setUsername($smtp->username);
$mailer->setPassword($smtp->password);
})
->to('[email protected]')
->send(new UserNotification());
}
This approach ensures emails are sent using the user's specific SMTP credentials without affecting other users or the default configuration.
Real-World Example 2: Switching Mail Providers Dynamically
Let's say your application routes emails differently based on their type. Transactional emails go through SendGrid, while marketing emails go through Mailgun.
Implementation:
You can dynamically change the SMTP details depending on the type of email:
use Illuminate\Support\Facades\Mail;
use App\Mail\TransactionalEmail;
use App\Mail\MarketingEmail;
public function sendTransactionalEmail()
{
Mail::mailer('smtp')
->build(function ($mailer) {
$mailer->setHost('smtp.sendgrid.net');
$mailer->setPort(587);
$mailer->setEncryption('tls');
$mailer->setUsername('sendgrid-username');
$mailer->setPassword('sendgrid-password');
})
->to('[email protected]')
->send(new TransactionalEmail());
}
public function sendMarketingEmail()
{
Mail::mailer('smtp')
->build(function ($mailer) {
$mailer->setHost('smtp.mailgun.org');
$mailer->setPort(587);
$mailer->setEncryption('tls');
$mailer->setUsername('mailgun-username');
$mailer->setPassword('mailgun-password');
})
->to('[email protected]')
->send(new MarketingEmail());
}
This pattern allows you to route emails dynamically while keeping your code modular and maintainable.
Real-World Example 3: Debugging and Testing
During development, you might want to test email functionality without affecting production users. Using Mail::build
, you can redirect emails to a testing SMTP server or simply log the messages.
Example: Using Mailtrap for Development
use Illuminate\Support\Facades\Mail;
use App\Mail\SampleEmail;
public function sendDebugEmail()
{
Mail::mailer('smtp')
->build(function ($mailer) {
$mailer->setHost('smtp.mailtrap.io');
$mailer->setPort(2525);
$mailer->setEncryption('tls');
$mailer->setUsername('mailtrap-username');
$mailer->setPassword('mailtrap-password');
})
->to('[email protected]')
->send(new SampleEmail());
}
This method ensures you don't accidentally send test emails to real users in production.
Best Practices for Runtime Mailers
-
Error Handling: Always validate and handle potential configuration errors (e.g., invalid credentials, connection failures).
try { Mail::mailer('smtp') ->build(function ($mailer) use ($smtp) { $mailer->setHost($smtp->host); $mailer->setPort($smtp->port); $mailer->setEncryption($smtp->encryption); $mailer->setUsername($smtp->username); $mailer->setPassword($smtp->password); }) ->to('[email protected]') ->send(new SomeMailable()); } catch (\Exception $e) { \Log::error('Mail sending failed: ' . $e->getMessage()); }
-
Secure Credentials: Always encrypt sensitive information like SMTP passwords before storing them in your database. Use Laravel's encryption utilities for this.
-
Logging: Log email events and configurations for debugging and auditing purposes.
-
Optimize Performance: Avoid building runtime mailers in performance-critical areas. Cache configurations where possible to reduce repetitive processing.
The ability to build runtime mailers with Mail::build
in Laravel offers a powerful toolset for handling dynamic email configurations. Whether it's user-specific SMTP settings, switching between email providers, or debugging in development, this feature allows you to adapt to a variety of real-world use cases.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
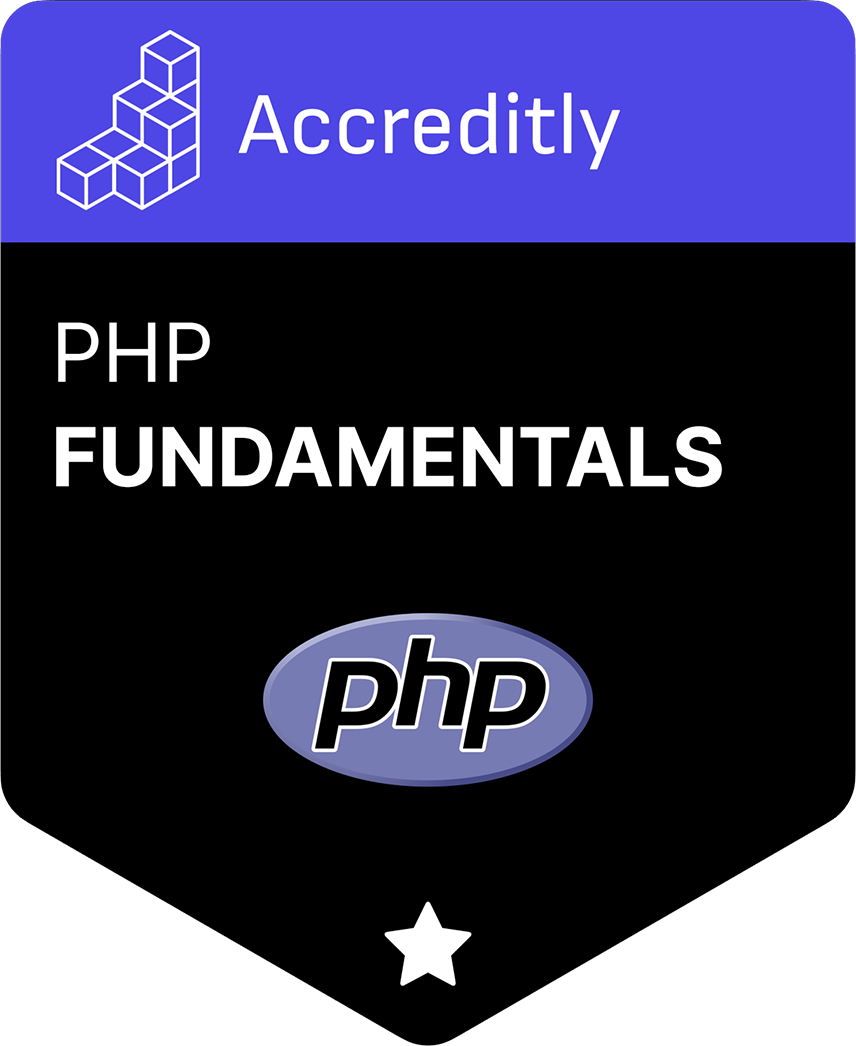