In JavaScript, the language's dynamic typing often leads to some interesting, and sometimes confusing, behaviors. One of these is the usage of the double not (!!) operator, which can seem mysterious if you're not familiar with it. Let's unpack it and see how it works.
The Not Operator
To understand the double not (!!
) operator, we first need to understand the single not (!
) operator. In JavaScript, !
is a logical operator that returns false if its single operand can be converted to true; otherwise, it returns true. It's essentially a way to perform a boolean negation. Here's an example:
console.log(!true); // Outputs: false
console.log(!false); // Outputs: true
The Double Not Operator
So what about the double not (!!
) operator? This isn't actually a separate operator in JavaScript, but rather two !
operators used together.
The first !
will convert the value to a boolean equivalent and negate it, and the second !
will negate it again. The net effect is that !!
will convert any value to its boolean equivalent.
console.log(!!"hello"); // Outputs: true
console.log(!!""); // Outputs: false
console.log(!!1); // Outputs: true
console.log(!!0); // Outputs: false
console.log(!!undefined); // Outputs: false
console.log(!!null); // Outputs: false
This is extremely useful because it allows you to take advantage of JavaScript's type coercion to quickly cast any value to its boolean equivalent.
Practical Use Case
One common use case for the !!
operator is to check if an element exists in the DOM:
let element = document.getElementById("element");
if (!!element) {
console.log("Element exists");
} else {
console.log("Element does not exist");
}
Here, !!element
will be true
if the element is found and false
if it's not.
While the double not (!!
) operator may seem unusual at first, it can be a powerful tool in your JavaScript toolkit once you understand its purpose. It allows for efficient and readable type coercion, ensuring any value can be properly treated as a boolean when needed. This operator, though simple, is a testament to the flexibility and utility of JavaScript as a dynamic programming language.
If you're interested in how the double not (!!
) operator works in other languages check out our article on using
the double not (!!
) operator in PHP.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
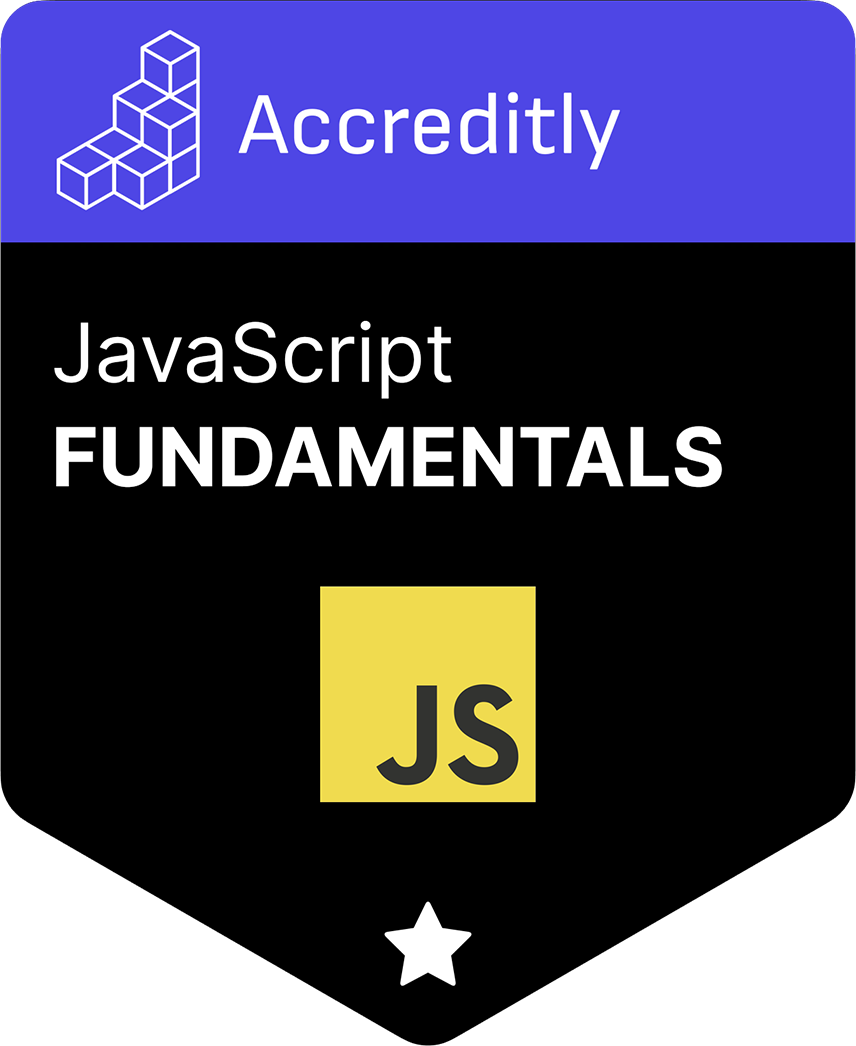